Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial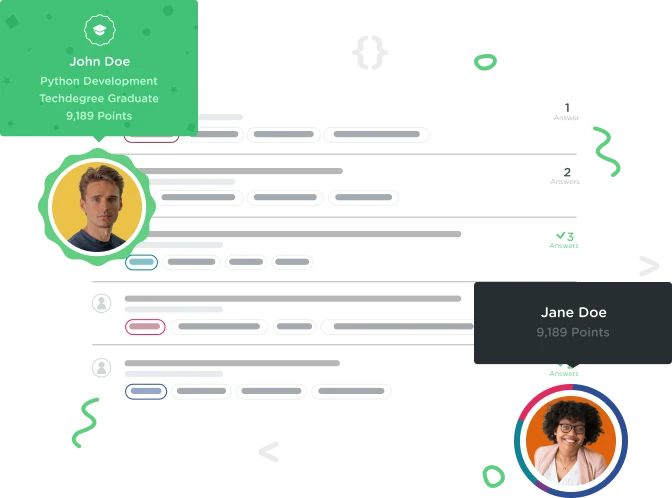

Vladimir Plokhotniuk
5,464 PointsWhy i cannot push selectively?
const teachers = [ { name: 'Ashley', topicArea: 'Javascript' } ]; let newTeachers = [ { name: 'James', topicArea: 'Javascript' }, { name: 'Treasure', topicArea: 'Javascript' } ];
function addNewTeachers(newTeachers) {
teachers.push(newTeachers); // working only teachers.push(...newTeachers);
}
addNewTeachers(newTeachers[0, 1]); //addNewTeachers(newTeachers);
4 Answers
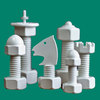
Steven Parker
229,786 PointsI'm not sure what you mean by "push selectively", but it looks like you're misunderstanding how the comma operator works in JavaScript:
As stated on the MDN documentation page:
The comma operator evaluates each of its operands (from left to right) and returns the value of the last operand.
So, "newTeachers[(0, 1)]
" is the same as "newTeachers[1]
".

Matthew Fung
8,643 PointsYou can selectively add items to an array, by manually adding them in the order you wish to add them. For instance:
const fruit = ['Apples' , 'Bananas', 'Oranges'];
const newFruit = ['Grapes', 'Pineapple', 'Melon'];
// I could either add a load of fruit at once, or only add specific fruit.
function addNewFruit (fruit) {
fruit.push(...newFruit); // This will iterate and push items in the newFruit array to the fruit array.
// RESULT: ['Apples' , 'Bananas', 'Oranges', 'Grapes', 'Pineapple', 'Melon']
}
function addSomeNewFruit (fruit) {
fruit.push(newFruit[0]); // Add the first item in the newFruit array to the fruit array
// RESULT: ['Apples' , 'Bananas', 'Oranges', 'Grapes']
fruit.push(newFruit[2]); // Add the third item in thew newFruit array to the fruit array
// RESULT: ['Apples' , 'Bananas', 'Oranges', 'Grapes', 'Melon']
}
This is fine for small arrays of items, but imagine if you had tens or hundreds of items! It's not common to want to add only certain items within an array to another one, but you could do it if you specify the index of the item you wish to add, rather than the entire array.
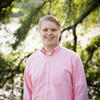
Josef Aidt
7,722 PointsIt looks as if your code allows you to "selectively" push a new teacher to the existing teachers
array of objects. If you are wanting to push the entire, new array of objects newTeachers
, then you'd have to iterate over each entry in the new array. Without iterating over each object in the new array, it will push the entire array as an entry into teachers
, which will end up looking like this:
[ { name: 'Ashley', topicArea: 'Javascript' },
[ { name: 'James', topicArea: 'Javascript' },
{ name: 'Treasure', topicArea: 'Javascript' } ] ]
To iterate over each entry in newTeachers
and insert into the existing teachers
array, you can use the Array.forEach()
method found here. Implementation of this method would look like this:
const teachers = [ { name: 'Ashley', topicArea: 'Javascript' } ];
let newTeachers = [ { name: 'James', topicArea: 'Javascript' }, { name: 'Treasure', topicArea: 'Javascript' } ];
newTeachers.forEach(i => {
teachers.push(i);
});
This will insert each object (in this case, teacher) into its own entry in the existing teachers
array.
[ { name: 'Ashley', topicArea: 'Javascript' },
{ name: 'James', topicArea: 'Javascript' },
{ name: 'Treasure', topicArea: 'Javascript' } ]

Vladimir Plokhotniuk
5,464 PointsIf we will have 5 teachers. How can i push 2 and 4 teacher, in example in the example above (i meant from the video, not like (...newTeachers), i need only 2 and 4? Thanks.
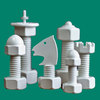
Steven Parker
229,786 PointsCan you explain what you mean by "2 and 4"? Your example has only 2 "newTeachers".
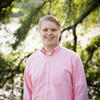
Josef Aidt
7,722 PointsYour code in your original post does just that. You will need to call the function multiple times to insert multiple records using the comma operator, exactly how you're doing it in your original post.

Vladimir Plokhotniuk
5,464 PointsSorry, if i explain wrong. If we have a lot of "newTeachers", but i need to push only second and fourth, in one line of code. https://w.trhou.se/ft7mytoxu9 see pls line 34
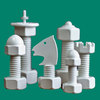
Steven Parker
229,786 PointsSee the example I added to my answer.
Vladimir Plokhotniuk
5,464 PointsVladimir Plokhotniuk
5,464 Pointsvar sports = ['soccer', 'baseball'];
var total = sports.push('football', 'swimming');
console.log(sports); // ['soccer', 'baseball', 'football', 'swimming']
...I had in mind an example like this.
And if we have an array of 20 elements, and need insert 8, and if they do not go in order ?
Steven Parker
229,786 PointsSteven Parker
229,786 PointsIn some cases, the order might not be important. But if it is, you control the order by how you add the items.
Steven Parker
229,786 PointsSteven Parker
229,786 PointsSo, for example, to push only the 2nd and 4th (indexes 1 and 3):
teachers.push(newTeachers[1], newTeachers[3]);