Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial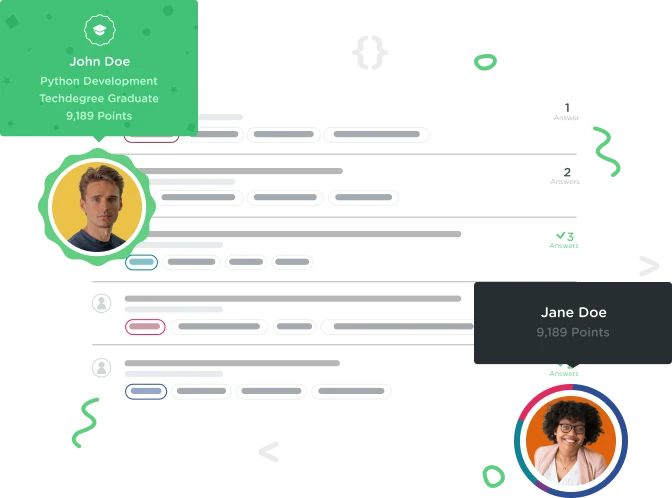
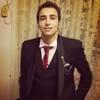
Ahmed Mohamed Fouad
11,735 Pointswhy I don't have to include Math Module inside a class to work it's methods, while I've to include Comparable method ?
why I don't have to include Math Module inside a class to work it's methods, while I've to include Comparable method to make it work
The Math Module will work either using include like here
class Examp
include Math
def to_s
puts Math::E # => 2.718281828459045
puts Math::PI # => 3.141592653589793
puts Math.sqrt(9) # => 3.0
puts Math.cos(1) # => 0.5403023058681398
puts Math.hypot(2, 2) # => 2.8284271247461903
puts Math.log(2, 10) # => 0.30102999566398114
puts Math.log(2, 12) # => 0.2789429456511298
end
end
exa = Examp.new
puts exa
or not using it like here
class Examp
def to_s
puts Math::E # => 2.718281828459045
puts Math::PI # => 3.141592653589793
puts Math.sqrt(9) # => 3.0
puts Math.cos(1) # => 0.5403023058681398
puts Math.hypot(2, 2) # => 2.8284271247461903
puts Math.log(2, 10) # => 0.30102999566398114
puts Math.log(2, 12) # => 0.2789429456511298
end
end
exa = Examp.new
puts exa
while the comparable module must be included to make the <=> method work like here
class Player
include Comparable
attr_accessor :name, :score
def <=>(other_player)
score <=> other_player.score
end
def initialize(name, score)
@name = name
@score = score
end
end
player1 = Player.new("Jason", 100)
player2 = Player.new("Kenneth", 80)
puts "player1 > player2: %s" % (player1 > player2)
puts "player1 < player2: %s" % (player1 < player2)
1 Answer
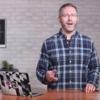
Jay McGavren
Treehouse TeacherA module in Ruby is just a collection of methods. Modules can be used in different ways. The way you are supposed to use it depends on the module.
Some modules (like Comparable
) are supposed to be added to a class with include
, so that the module's methods become instance methods of the class.
Other modules (like Math
) are meant to be used all by themselves. You call methods directly on the module itself.
The documentation for the module will usually show you how the module is supposed to be used. Here's the documentation for Comparable
. Here's the documentation for Math
.