Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial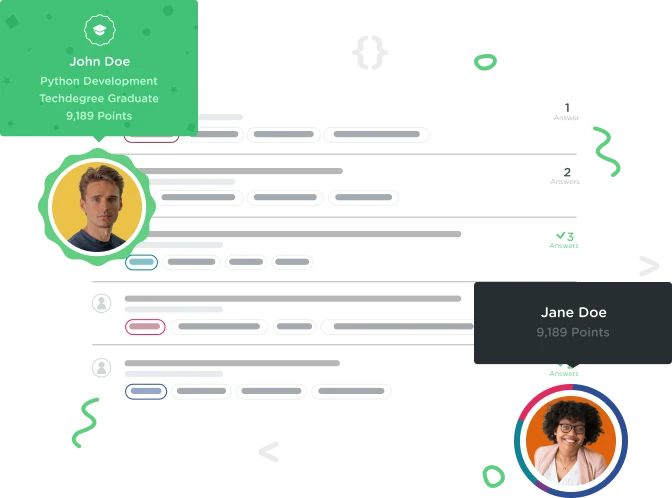
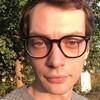
Tomasz Grodzki
8,130 PointsWhy I get info that setter method returns a wrong value?
Everything should be fine. I passed a level to setter method. Then there are conditional statements, which are fine. And I return a value of level or 'None' (depends on students level).
So why the heck it tells me that I return a wrong value?
I added two last lines just to show that code works fine. But anyway I still get a bummer.
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
set major ( level ) {
if ( level === 'Senior' || level === 'Junior' ) {
this._major = level;
} else {
this._major = 'None';
}
}
}
var student = new Student(3.9, 60);
student.major = student.level;
console.log ( student );
5 Answers

jhon white
20,006 PointsBecause of (level) is not (this.level) . They are different variables . if you wanna see the differences add this in your code:
student.major='Junior'; out put of your console.log statement:
console.log ( level: ${level} and this.level: ${this.level}
)
will be:
level: Junior and this.level: Senior
and you get the same log message for both code because you log(this) which is the class instant with same input.

jhon white
20,006 Pointsset major(major) { this._major = major if (this.level !== 'Senior' && this.level !== 'Junior') { this._major = 'None' } }
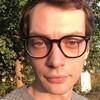
Tomasz Grodzki
8,130 PointsYour code actually does work. But is there any reason why mine doesn't? Is there any mistake in my code? I think that these codes are functionally the same, aren't they?

jhon white
20,006 PointsYou didn't set _major to the paramater of the function before chacking the level. also when you have if and else statement you can make it shorter.
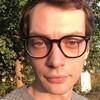
Tomasz Grodzki
8,130 PointsBut why should I? Is it mistake?
In the console the student student object looks fine after recall student.major = student.level.

jhon white
20,006 Pointsyour variables name confused the function. your input and your instant variable are the same, so you should use (this) key word in your condition. set major (level ) { if (this. level === 'Senior' || this.level === 'Junior' ) { this._major = level; } else { this._major = 'None'; } }
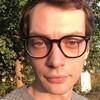
Tomasz Grodzki
8,130 PointsI completely don't get it. Well... I already can write a code that passes this test... But I don't get it.
Look at this, I wrote 2 codes. First one does pass, and the second one doesn't. Why is that? I checked both variants, and according to console log, there is no difference between this.level and level. Both variants shows me the result! It's too confusing.
I thought like you wrote, that I confused variables by wrong using this keyword... But according to console log, there's no difference! Could you explain this, or give me some materials that'll helps me to understand this crazy shit?
First code:
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
set major ( level ) {
if ( this.level === 'Senior' || this.level === 'Junior' ) {
console.log ( `level: ${level} and this.level: ${this.level}`)
this._major = level;
} else {
this._major = 'None';
}
console.log ( this );
}
}
var student = new Student(3.9, 100);
student.major = student.level;
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
set major ( level ) {
if ( level === 'Senior' || level === 'Junior' ) {
console.log ( `level: ${level} and this.level: ${this.level}`)
this._major = level;
} else {
this._major = 'None';
}
console.log ( this );
}
}
var student = new Student(3.9, 100);
student.major = student.level;

jhon white
20,006 PointsYou are welcome. Good Luck :)
Tomasz Grodzki
8,130 PointsTomasz Grodzki
8,130 PointsThank you thank you thank you. Now I see the point. You were very helpful!