Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial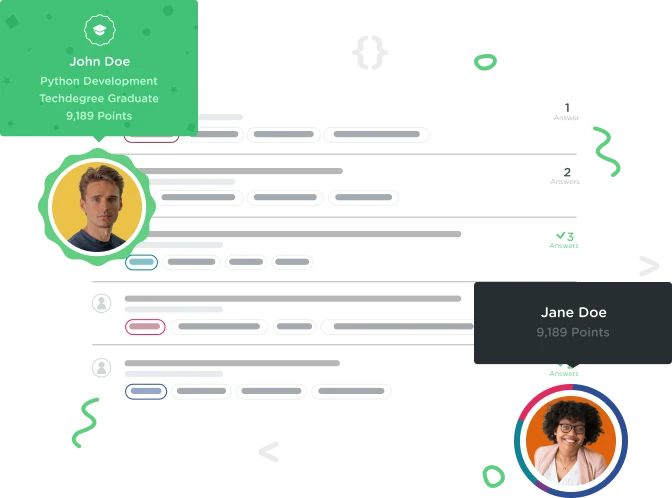

Unsubscribed User
11,042 PointsWhy I need to pass the todo_list when I have the todo_list method defined with let?
I can't figure out why we still pass the todo_list obj to the update_todo_list method... We already have a "todo_list" memoized method! Please have a look at the comments I wrote :-)
require 'spec_helper'
describe "Editing todo lists" do
let!(:todo_list) { TodoList.create(title: "Groceries", description: "Grocery list.") }
def update_todo_list(options={})
options[:title] ||= "My todo list"
options[:description] ||= "This is my todo list."
#WE USED LET, WHY THIS STATEMENT?
todo_list = options[:todo_list]
visit "/todo_lists"
within "#todo_list_#{todo_list.id}" do
click_link "Edit"
end
fill_in "Title", with: options[:title]
fill_in "Description", with: options[:description]
click_button "Update Todo list"
end
it "updates a todo list successfully with correct information" do
update_todo_list todo_list: todo_list,
title: "New title",
description: "New description"
todo_list.reload
expect(page).to have_content("Todo list was successfully updated")
expect(todo_list.title).to eq("New title")
expect(todo_list.description).to eq("New description")
end
it "displays an error with no title" do
#WE USED LET, WHY PASS THE todo_list OBJECT?
update_todo_list todo_list: todo_list, title: ""
title = todo_list.title
todo_list.reload
expect(todo_list.title).to eq(title)
expect(page).to have_content("error")
end
it "displays an error with too short a title" do
update_todo_list todo_list: todo_list, title: "hi"
expect(page).to have_content("error")
end
it "displays an error with no description" do
update_todo_list todo_list: todo_list, description: ""
expect(page).to have_content("error")
end
it "displays an error with too short a description" do
update_todo_list todo_list: todo_list, description: "hi"
expect(page).to have_content("error")
end
end
1 Answer
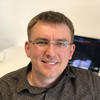
Ilya Dolgirev
35,375 PointsThe idea of this spec is to test whole feature and not the model and its methods itself.
This helper method update_todo_list
is using Capybara to imitate real user interaction with the page.
That is why you have to clearly define the todo object you're trying to update because in real app you'll have many lists as user.