Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial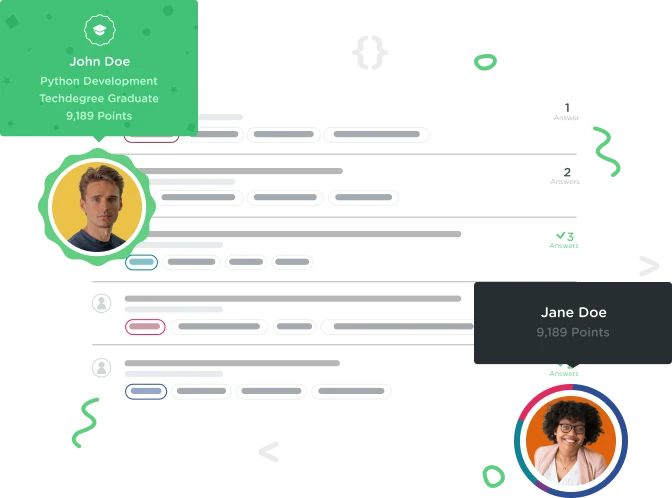

Voon Zhi
3,827 PointsWhy interface is so important since we can still perform the same function of a class without implementing it?
PHP Object Oriented Programming
1 Answer
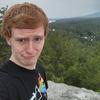
Brendon Butler
4,254 PointsI program in Java, so things may not translate directly to PHP. I'm going to try to write the most simplistic Java I can.
Interfaces are great because they allow you to set specific parameters that need to be met for a class to function. Then you can have classes that implement this interface so that it takes in all its default methods.
Lets say you're making a game, and you have an interface named "Humanoid."
interface Humanoid {
// here's a default method to return a name for this humanoid.
public String getName();
// get the humanoid's health.
public int getHealth();
// set the humanoid's health.
public void setHealth(int amount);
}
Now you want to have 2 human-like objects. Let's say a Skeleton and a Zombie. If you implement the "Humanoid" interface, you have to include the methods pre-defined by said interface.
class Zombie implements Humanoid {
String name; // class level name variable
int health; // class level health variable
// constructor method (for creating a new Zombie)
Zombie (String humanoidName, int healthAmount) {
name = humanoidName;
health = healthAmount;
}
// implementing the "getName()" method from the Humanoid interface. Now we must fill out the details.
public String getName() {
return name;
}
public int getHealth() {
return health;
}
public void setHealth(int amount) {
health = amount;
}
}
class Skeleton implements Humanoid {
String name;
int health;
Skeleton (String humanoidName, int healthAmount) {
name = humanoidName;
health = healthAmount;
}
public String getName() {
return name;
}
public int getHealth() {
return health;
}
public void setHealth(int amount) {
health = amount;
}
}
Now we're going to create some entities and manipulate them. Hopefully this is where the importance will start to make sense.
class Main {
public static void main() {
//lets start off by making a zombie and a skeleton
Zombie zombie1 = new Zombie("Bob Zombie", 20);
Skeleton skeleton1 = new Skeleton("Skelly", 10);
// lets use the method below (isZombie) to tell whether or not we have a zombie
isZombie(zombie1); // output would be "true"
isZombie(skeleton1); // output would be "false"
// see, you can use either class type in this method without having to create two separate methods, saves you time and code length
// we can also do damage to these entities using the "hit" method below
zombie1.getHealth(); // health = 20
hit(zombie1);
zombie1.getHealth(); // health = 18
// now lets create another Zombie and another Skeleton, but instead of using their actual class types, we'll allow for manipulation by using their interfaces
Humanoid humanoid1 = new Zombie("Brains", 12);
Humanoid humanoid2 = new Skeleton("Bones", 5);
}
// if you want a method that could take any class that implements your interface, you can use the interface as a variable
public boolean isZombie(Humanoid humanoid) {
if (humanoid.instanceOf(Zombie))
return true; // returns true if the inputted class is a Zombie class
return false; // returns false if the inputted class is NOT a Zombie class (for example, a Skeleton class)
}
public boolean hit(Humanoid humanoid) {
humanoid.setHealth(humanoid.getHealth() - 2);
}
}
Hopefully this translates well enough and the comments aren't too confusing, I tried my best. Interfaces are also helpful if you create a program that allows for plugins. You can set which methods are necessary and your program would only have to know what is needed by the interface. That way people wouldn't have to go into your code and modify things to add a new feature or something.
Sure you can do all these things without interfaces, but from my experience, it makes things a lot easier!