Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial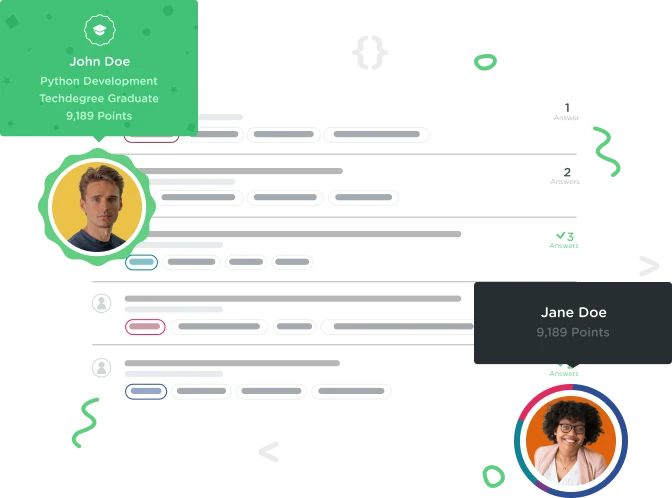

Richard Fritts
3,145 PointsWhy introduce second variable for same calculation?
def split_check(total, number_of_people):
cost_per_person = total / number_of_people
return cost_per_person
cost_per_person = split_check(84.97, 4)
print ("Each person owes ${}".format(cost_per_person))
Why create new variable amount_due on line 5 and line 6? Why not continue using cost_per_person as was done on lines 2 and 3?
[MOD: added ```python formatting -cf]
1 Answer
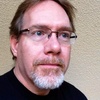
Chris Freeman
Treehouse Moderator 68,441 PointsHey Richard Fritts, this is an excellent question! It helps illustrate how Python passes object references and variable names are merely labels for those references.
Inside the function, a float
object is created. A reference variable name cost_per_person
is assigned to point at that object. The return
statement returns a reference to the float object.
The function call results in an object reference: the same one returned by the function return
statement. The reference variable name outside the function is assigned to point at this returned object reference.
So regardless of the name inside or outside the function, the two references point at the same object! In the code below, the object id (location in memory) is the same for both variables. There is only one object. Variable names are cheap, so making them match may only serve readability.
def split_check(total, number_of_people):
cost_per_person = total / number_of_people
print("inside function var id is: ", id(cost_per_person))
return cost_per_person
cost_per_person2 = split_check(84.97, 4)
print("outside function var id is: ", id(cost_per_person2))
print("Each person owes ${}".format(cost_per_person2))
# inside function var id is: 5828415728
# outside function var id is: 5828415728
# Each person owes $21.2425
Post back if you need more help. Good luck!!!