Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial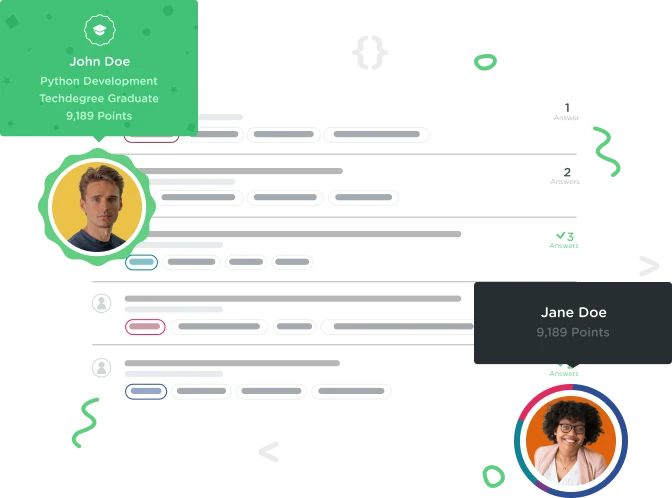

nico dev
20,364 PointsWhy is a callback and a variable assignment needed here, instead of testing the function straightaway?
Hi community,
I would appreciate today receiving some insight, because I dealt with this challenge for some while, and although I could finally solve it, I can't quite wrap my head around why, or learn from it, but I'd like to.
First this is the base code:
function subtraction (number1, number2) {
if (typeof number1 !== 'number' || typeof number2 !== 'number') {
throw Error('subtraction only works with numbers!')
}
return number1 - number2
}
This code is in some wherever
module (the path doesn't really count because it's just a challenge anyway).
Now the challenge's code is:
var expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('only works with numbers', function () {
// YOUR CODE HERE
})
})
And the idea of the challenge is that we can test (and prove) that the function will throw an error (our customized error, specifically) when passed any NaN as an argument.
Now, I was trying to do it this way (among others):
describe('subtraction', function () {
var subtraction = require('../game_logic/subtraction.js').subtraction
it('only works with numbers', function () {
// YOUR CODE HERE
var num = 45
var letters = 'Hello'
expect(subtraction(num, letters)).to.throw('subtraction only works with numbers!')
})
})
// Also tried other options, like:
// var num = 45
// var letters = 'Hello'
// var result = subtraction(num, letters)
// expect(result).to.throw('subtraction only works with numbers!')
But all of these would return an error in the Mocha tests stating:
TypeError: subtraction is not a function
.
Now, when I tried to do it with a callback with the function inside, being assigned to a variable, more like we did in the last video, it worked:
var expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('only works with numbers', function () {
// YOUR CODE HERE
var num = 45
var letters = 'Hello'
var result = function() {
subtraction(num, letters)
}
expect(result).to.throw('subtraction only works with numbers!');
})
})
The truth is I don't fully understand why this behavior? Isn't passing the function giving it the return value (or in this case the error thrown instead) to be tested? Just fighting to understand that bit.
Thanks in advance for any insight, suggestion, idea, correction, or alike about it!
1 Answer
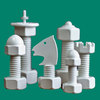
Steven Parker
241,809 PointsThe difference is in what you are passing to the expect function, and when the function being tested gets called. For example, in this code:
expect(subtraction(num, letters)).to.throw('subtraction only works with numbers!')
The function subtraction will be called first, and after it runs, the result value it returns will be supplied as the argument to expect. Any exception thrown will apply to the outer context (the "it" context) before expect is called.
On the other hand, in this code:
expect(result).to.throw('subtraction only works with numbers!');
The function result is itself passed as the argument to expect, which will call it from within its internal context and check that it throws the exception.
If you didn't want to create the intermediate function and variables, you could also do this:
// YOUR CODE HERE
expect(subtraction.bind(null, 45, 'Hello')).to.throw('subtraction only works with numbers!');
nico dev
20,364 Pointsnico dev
20,364 PointsThere you go! Sure, it clicked now on me. It is some kind of closure mechanism somehow, right? If I will pass it the function to the expect itself, then it will only give the return to expect, but if I pass the variable, which contains the function, then it will give the whole function (with whatever it produces, returns, errors and all) to the expect, yes?
Very clear now, Steven, thanks for your explanation and examples! You always nail it! :) At least with me, when I read you, I wrap my head around the tough ones.