Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial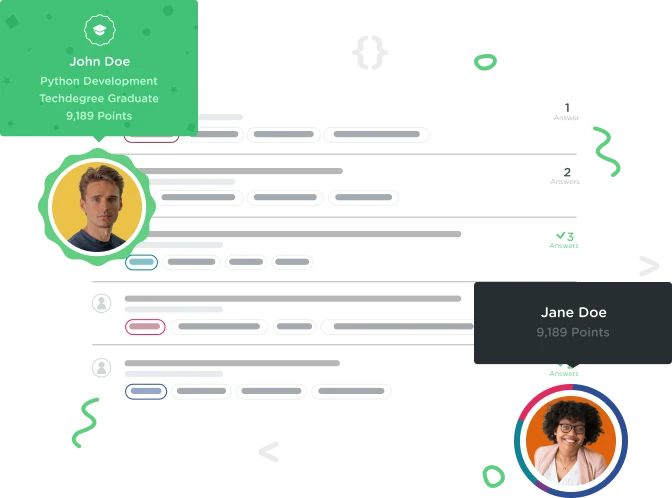
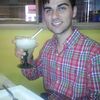
Nicholas Lee
12,474 PointsWhy is an error thrown, color is not defined?
In the following code, what will be output by the console.log call?
function example ( ) {
var color = "red";
return color;
}
example("blue");
console.log(color);
Why is "blue" not passed though the parameters as a new argument?
2 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsThe color
variable is defined for the scope of the example
function. It does not exist outside of the function, so that's why the console.log
results in a reference error.
The string "blue"
is passed to the function as a parameter, but you're not doing anything with it inside the function. Also, your function doesn't have a named parameter so the only way to access it would be through the arguments
Array-like object.
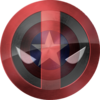
J Scott Erickson
11,883 PointsThe global scope doesn't have access to the color variable that you have defined in the function. Unless you are missing some code where here is another 'color' variable defined anything outside of the scope of the function doesn't have any 'visibility' or 'knowledge' of the color variable.
This:
example("Blue");
Doesn't actually do anything, as the function definition does not have any arguments accepted and doesn't set the color variable based on a passed argument.
console.log(color);
will throw a reference error because, as I stated before, color is a variable only in the scope of the example() function and console has no visibility into it.
Jim Withington
12,025 PointsJim Withington
12,025 PointsThis is from one of the JavaScript Foundations quizzes, just so you know. :-)
My follow-up: if we adjusted it like this, how come the console.log here doesn't display anything?
Is there a way to get the console to display that color?