Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial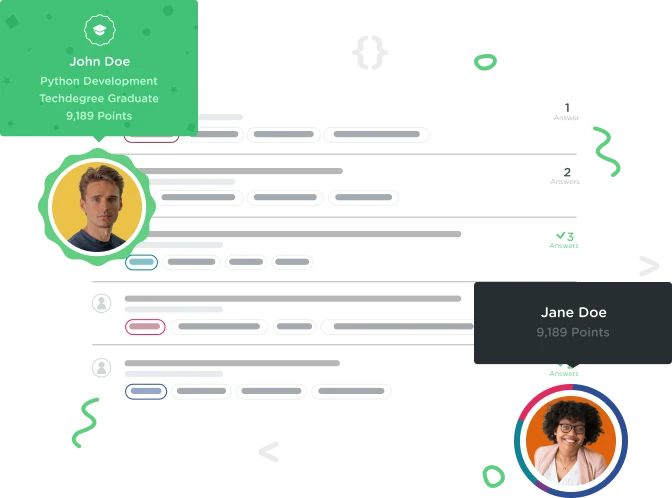
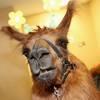
A X
12,842 PointsWhy is code sometimes read from left to right and then others from right to left?
using System;
namespace Treehouse.FitnessFrog
{
class Program
{
static void Main()
{
int runningTotal = 0; /*read left to right: we're making an int variable called runningTotal and assigning it the value 0 */
Console.Write("Enter how many minutes you exercised: "); //read left to right
string entry = Console.ReadLine(); //read left to right
int minutes = int.Parse(entry); //read RIGHT side of the equals sign to LEFT?
runningTotal = runningTotal + minutes; //read the RIGHT side of the equals sign to LEFT for reasons unclear to me
Console.WriteLine("You've entered " + runningTotal + " minutes."); //read left to right
}
}
}
Jeremy discusses in the video this is referencing that runningTotal = runningTotal + minutes; is read from right side of the equals sign to the left, but I'm not clear why we're suddenly interpreting the code from right to left vs. left to right like all the other code we've dealt with so far. This is confusing, and I'm unclear what circumstances are read right to left vs. left to right. Can someone clear this up for me? Thanks!
2 Answers

Simon Coates
28,694 Pointsok, keep in mind i really don't know what i'm talking about, but I think it might be a function of operators. Operator precedence determines which bits get evaluated first, and in some languages at least, the operators seems to come with an idea of direction to read. I had a quick google and saw this article on c#, which might be on topic: https://msdn.microsoft.com/en-us/library/aa691323(v=vs.71).aspx

Avan Sharma
7,652 PointsPrecedence of Operators. In case of assignment operation, always right side of the code is calculated first and is assigned to left side.
int a = 4; Here 'a' is declared first and value of 4 is assigned from right side to left.
Steven Parker
231,275 PointsSteven Parker
231,275 Points