Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial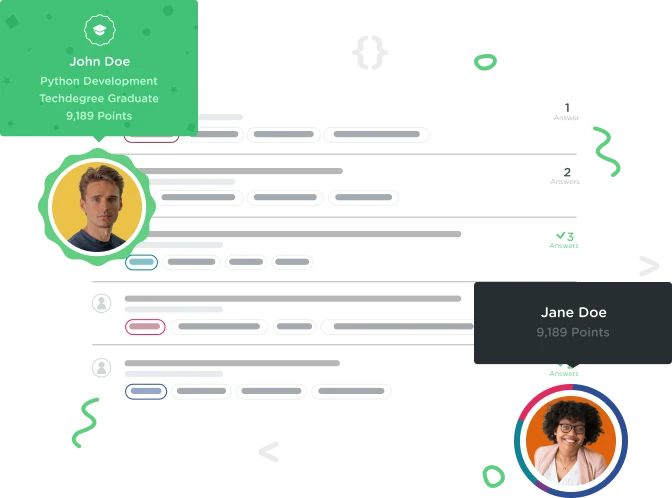

Youssef Moustahib
7,779 PointsWhy is Counter() not working?
I did this code the long way at first, then I saw the Counter() solution posted elsewhere. Why is it not working?
1) I converted x into a list to iterate through the members 2) I lowercased the letters 3) I used counter to insert what the question wanted into my new 'end' variable 4) I returned end.
Please help. Thanks!
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
from collections import Counter
def word_count(x):
x = x.split()
x = x.lower()
end = Counter(x)
return end
2 Answers
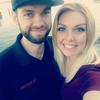
Bryan Reed
11,747 PointsYou want to lowercase the string before you split it. You can trail the functions together to make it a bit easier as well:
from collections import Counter
def word_count(x):
x = x.lower().split()
end = Counter(x)
return end

Nathan Parsons
16,402 PointsI think your issue is that when use .split() on the string x you get back a list, but you cannot call .lower() on lists, only strings. At the time you call .lower(), your x variable is a list of strings and is no longer a string object. Try to call .lower() on your string first to get a lower case string, then split that lower case string into a list and call Counter.
In short, swap the order in which you call .split() and .lower().