Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial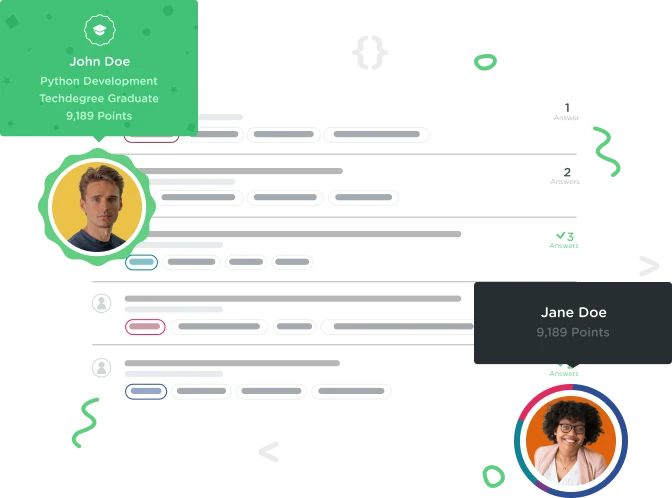

Henry Williams
2,889 PointsWhy is create_row returning so many cards?
AFAIK my code is the same for the create_row method as in the video:
from cards import Card
import random
class Game:
def __init__(self):
self.size = 4
self.card_options = ['to', 'yo', 'fi', 'fo',
'mo', 'it', 'ab', 'ta']
self.cards = []
self.columns = ['A', 'B', 'C', 'D']
self.locations = []
for column in self.columns:
for num in range(1, self.size + 1):
self.locations.append(f'{num}{column}')
def set_cards(self):
used_locations = []
for word in self.card_options:
for i in range(2):
available_locations = set(self.locations) - set(used_locations)
random_location = random.choice(list(available_locations))
used_locations.append(random_location)
card = Card(word, random_location)
self.cards.append(card)
def create_row(self, num):
row = []
for column in self.columns:
for card in self.cards:
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append(' ')
return row
if __name__ == '__main__':
game = Game()
game.set_cards()
game.cards[0].matched = True
game.cards[1].matched = True
game.cards[2].matched = True
game.cards[3].matched = True
print(game.create_row(1))
print(game.create_row(2))
print(game.create_row(3))
print(game.create_row(4))
but this is what I return:
[' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',
' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '
', ' ', ' ', ' ', ' ', ' ', ' ', ' ']
[' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',
' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '
', ' ', ' ', ' ', ' ', ' ', ' ', ' ']
[' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',
' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '
', ' ', ' ', ' ', ' ', ' ', ' ', ' ']
[' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',
' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '
', ' ', ' ', ' ', ' ', ' ', ' ', ' ']
What's going wrong here?
2 Answers

jb30
44,807 PointsIn create_row
,
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append(' ')
The code currently appends ' '
to row
whenever card.location == f'{column}{num}
is false. Try increasing the indent of the else block so that it is at the same level as if card.matched:
.
if card.location == f'{column}{num}':
if card.matched:
row.append(str(card))
else:
row.append(' ')
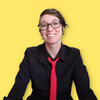
Mel Rumsey
Treehouse ModeratorHey Henry Williams It looks like your code does match Megan's other than your word sizes are 2 rather than 3. The issue might be in your cards.py
file. Are you able to send a snapshot link of your workspace so that I can dig a bit further in the code?

Henry Williams
2,889 PointsHowdy Mel, I thought that might be the culprit too so I took a look back and forth and my beginner's eyes just aren't seeing what's off.
Henry Williams
2,889 PointsHenry Williams
2,889 PointsThat was it. I remember I had inadvertently changed the indent size only to go back later and try and correct it...and that slipped out of place. Thanks!