Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial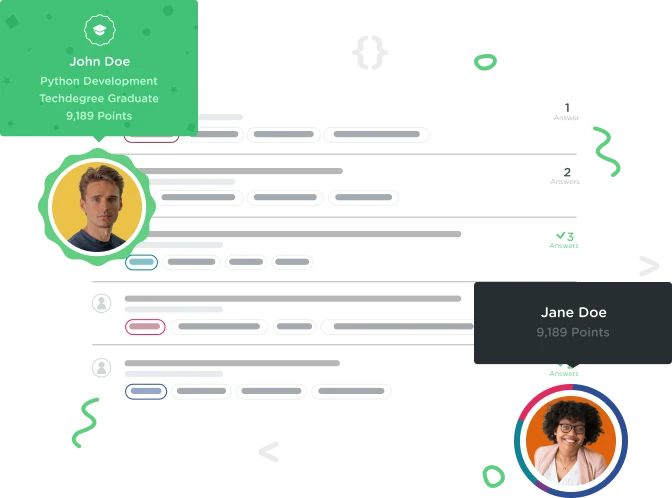

Peter Byriel
9,643 PointsWhy is extend not working with range() here?
the_list.extend(range(1, 21))
- works fine in python in terminal, but not here?
16 Answers
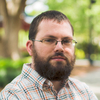
Kenneth Love
Treehouse Guest TeacherOK, let's step through it.
- Step 0:
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
- Step 1:
the_list.insert(0, the_list.pop(3))
the_list
is now[1, "a", 2, 3, False, [1, 2, 3]]
- Step 2:
the_list.remove('a')
the_list.remove(False)
the_list.remove([1, 2, 3])
the_list
is now [1, 2, 3]
- Step 3: Make
the_list
have all of the numbers from 1 to 20.
I'll leave the rest to you.

Ryan Arthur
4,910 PointsHere's what I came up with
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.pop(3)
the_list.insert(0, 1)
the_list.remove([1, 2, 3])
del the_list[1]
del the_list[3]
the_list.extend(range(4,21))
Quite a mental approach I must say, but it works!

Tony McCabe
4,889 PointsThank You!
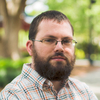
Kenneth Love
Treehouse Guest TeacherIt'll .extend()
the list with the range()
just fine. The problem is the contents of your the_list
before and after the .extend()
. It should be 1-20 with each number only appearing once...what do you have?

Peter Byriel
9,643 PointsAh, get it now!
Suggestion: Rephrase the text to "Make the list contain ONLY the numbers 1 to 20 using extend()" That would make it much clearer that it's not just a matter of making the list contain the numbers (which extend(1, 21) does), but of making sure 1-20 is all it contains.
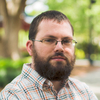
Kenneth Love
Treehouse Guest TeacherDone. Hopefully that helps future students get through it more smoothly.
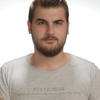
Erdem Meral
25,022 Pointsthe_list = ["a", 2, 3, 1, False, [1, 2, 3]]
the_list.insert(0, the_list.pop(3)) # the_list is now [1, "a", 2, 3, False, [1, 2, 3]]
the_list.remove('a')
the_list.remove(False)
the_list.remove([1, 2, 3])
the_list.extend(range(4,21))
these are my codes why its not working? i had already 1 2 3 in my list so i just add the rest
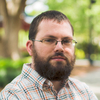
Kenneth Love
Treehouse Guest TeacherI just tried it and it passed for me. Are you getting an error message that you can share?

Jean Beltre
1,181 PointsI was getting an error here as well
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.insert(0, the_list.pop(3))
list=[5, 4, 1]
for numbers in list:
del the_list[numbers]
the_list.extend(range(4,21))
print(the_list)
Error: Traceback (most recent call last): File "4fd7aca7-edd9-4f26-bb1e-8f7ba011333e.py", line 105, in """) File "", line 2, in TypeError: 'list' object is not callable
I tested the script in workspace and it works fine for me.

Anthony Attard
43,915 PointsYou can just reset the list right before adding the numbers.
the_list = []
the_list.extend(range(1, 21))

Peter Byriel
9,643 Points''' x = the_list.pop(3) the_list.insert(0,x) del the_list[1] del the_list[3] del the_list[3] '''
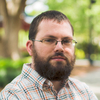
Kenneth Love
Treehouse Guest TeacherRight, so at that point, what is the content of the_list
?

Peter Byriel
9,643 PointsWill need to work that out - but the code passed the prior assignment so I didn't think much about that :-)

Peter Byriel
9,643 PointsThis doesn't work either - so I'm thinking it's a bug?
x = the_list.pop(3)
the_list.insert(0,x)
the_list.remove("a")
the_list.remove(False)
the_list.remove([1, 2, 3])
the_list.extend(range(1,21))

Tony McCabe
4,889 PointsMUZ140330 Simbarashe Chibgwe is correct. it worked for me when I placed my code where it say's # Your code goes below here:
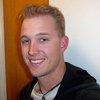
Richard Boag
5,547 PointsHow do you post your code so it looks like that? I tried the ''' method from the markdown cheatsheet, but it doesn't work. Any tips? My code looks exactly like you guys' but it's saying Task 1 is no longer passing.
Here's my code:
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.insert(0, the_list.pop(3))
the_list.remove("a")
the_list.remove(False)
the_list.remove([1, 2, 3])
the_list.extend(range(4:21))
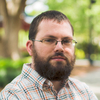
Kenneth Love
Treehouse Guest TeacherYou need to use three backticks (on a US keyboard, they're to the left of the 1) to get the code block. You can follow it with a language name to get syntax highlighting.
As for why your code isn't working, you need a comma in your range()
call, not a colon.
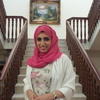
Assma Al-Adawi
8,723 PointsIs there no way to remove all items in one step? E.g. the_list.remove("a", False, [1, 2 ,3])??

Jonathon Mohon
3,165 PointsI used a list comprehension to do it like this:
the_list = [int(x) for x in the_list if str(x).isdigit()]
The int(x) is referring to the list item and I am casting it to an integer. For x is the iterator, in the_list is what its iterating through and the test condition is the if str(x) which is the current list item is a digit or not. If false it is not reassigned to the new list, if true it is. So this builds a new list and filters out all non numbers.
I had to use str(x) in the condition because isdigit is a string method. I used the int(x) in the start so that if there was a number being represented as a string: '2' it wouldn't be placed in the new list as a string.
I have been hanging out here: https://discord.gg/d39VM asking questions about Python and getting help.
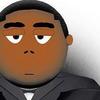
Joshua Howard
13,615 PointsHi Guys,
I actually figured this out by creating another list. There are better ways to do this, but it was get you past the test.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
the_list2 = [4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20]
# Your code goes below here
p = the_list.pop(3)
s = the_list.insert(0, p)
the_list.remove("a")
the_list.remove(False)
the_list.remove([1, 2, 3])
the_list.extend(the_list2)
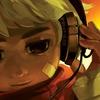
Jamal Scott
9,656 Pointsthe_list = ["a", 2, 3, 1, False, [1, 2, 3]]
index = the_list.pop(3)
the_list.insert(0, index)
the_list.remove("a")
del the_list[3]
del the_list[3]
print (the_list)
#checks and loops until the last number in the list reaches 20
while the_list[-1] != 20:
#add 1 to the last number in the list every time it loops and append it to the_list
the_list.append(the_list[-1] + 1)
print (the_list)
if anyone needs help this is the solution, but be sure to read through and understand
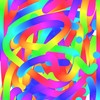
MUZ140330 Simbarashe Chibgwe
2,825 Pointsthe_list = ["a", 2, 3, 1, False, [1, 2, 3]]
Your code goes below here
the_list.insert(0, the_list.pop(3))
the_list.remove("a") the_list.remove(False) the_list.remove([1,2,3])
the_list.extend(range(3, 21))
MUZ140330 Simbarashe Chibgwe
2,825 PointsMUZ140330 Simbarashe Chibgwe
2,825 PointsI think it's including other elements like 1,2,3 in the_list. So it's duplicating instead of extending elements 4 to 20.
try
the_list.extend(range(4, 21))