Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial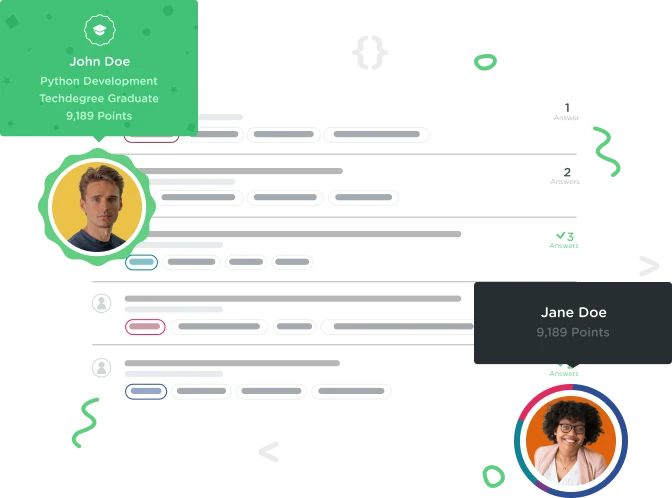

Joan Ang
784 PointsWhy is header("Location: contact.php?status=thanks"); redirect not working?
The "contact.php" page loads fine, but when I submit the form, the address remains "contact.php", and doesn't load (this is because of exit
?). I'm expecting the address to change to "contact.php?status=thanks".
If I change <form method="post" action="contact.php">
to <form method="post" action="contact-process.php">
it works fine.
Stackoverflow says it has something to do with unintentional white space but I still haven't figured out how to fix it.
header() must be called before any actual output is sent, either by normal HTML tags, blank lines in a file, or from PHP http://stackoverflow.com/questions/12525251/header-location-not-working-in-my-php-code
Here's my code for "contact.php":
<?php
if ($_SERVER["REQUEST_METHOD"]=="POST"){
$name = $_POST["name"];
$email = $_POST["email"];
$message = $_POST["message"];
$email_body ="";
$email_body = $email_body . "Name: " . $name . "\n";
$email_body = $email_body . "Email:" . $email . "\n";
$email_body = $email_body . "Message: " . $message;
// TODO: Send Email
header("Location: contact.php?status=thanks");
exit;
}?><?php
$pageTitle = "Contact Mike";
$section = "contact";
include('inc/header.php');?>
<div class ="section page">
<div class="wrapper">
<h1> Contact</h1>
<?php if (isset($_GET["status"]) AND $_GET["status"]=="thanks") { ?>
<p>Thanks for your message!</p>
<?php } else { ?>
<p>I'd love to hear from you! Complete the form to send me an email.</p>
<form method="post" action="contact.php">
<table>
<tr>
<th>
<label for="name"> Name</label>
</th>
<td>
<input type="text" name="name" id="name">
</td>
</tr>
<tr>
<th>
<label for="email"> Email</label>
</th>
<td>
<input type="email" name="email" id="email">
</td>
</tr>
<tr>
<th>
<label for="message"> Message</label>
</th>
<td>
<textarea name="message" id="message" placeholder="Leave me a message!"></textarea>
</td>
</tr>
</table>
<input type="submit" value="send">
</form>
<?php } ?>
</div>
</div>
<?php include('inc/footer.php'); ?>
And for contact-process.php:
<?php
$name = $_POST["name"];
$email = $_POST["email"];
$message = $_POST["message"];
$email_body ="";
$email_body = $email_body . "Name: " . $name . "\n";
$email_body = $email_body . "Email:" . $email . "\n";
$email_body = $email_body . "Message: " . $message;
// TODO: Send Email
header("Location: contact.php?status=thanks");
exit;
?>
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Joan,
I don't know if there's any kind of tool that can automatically check for this. I think you have to just look over your code to see where you are outputting something.
Since this has happened to you now with the extra whitespace before your opening php block you'll probably remember to check for that from now on.
Here's the header
page from the php manual which gives you other things to look out for: http://php.net/manual/en/function.header.php
Another thing to watch out for is saving your files as utf-8 with BOM. This produces output at the beginning of the file.
http://stackoverflow.com/questions/10199355/php-include-causes-white-space-at-the-top-of-the-page

Joan Ang
784 PointsTook forever, but finally found the extra whitespace at the top of my file.
Is there a good way for looking for this kind of thing?