Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial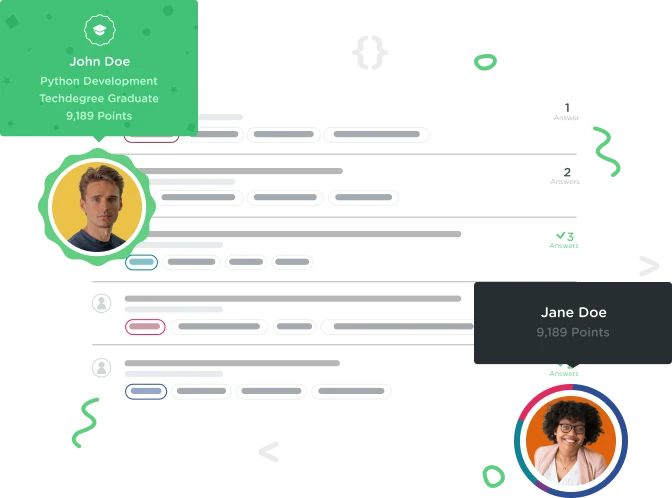
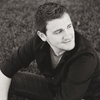
Zachary Zell
4,747 PointsWhy is inputted name not showing up?
package com.zachary.interactivestory.ui;
import android.content.Intent; import android.graphics.drawable.Drawable; import android.media.Image; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.util.Log; import android.widget.Button; import android.widget.ImageView; import android.widget.TextView;
import com.zachary.interactivestory.R; import com.zachary.interactivestory.model.Page; import com.zachary.interactivestory.model.Story;
import org.w3c.dom.Text;
public class StoryActivity extends ActionBarActivity {
public static final String TAG = StoryActivity.class.getSimpleName();
private Story mStory = new Story();
private ImageView mImageView;
private TextView mTextView;
private Button mChoice1;
private Button mChoice2;
private String mName;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story);
Intent intent = getIntent();
String mName = intent.getStringExtra(getString(R.string.key_name));
if (mName == null) {
mName = "Friend";
}
Log.d(TAG, mName);
mImageView = (ImageView)findViewById(R.id.storyImageView);
mTextView = (TextView)findViewById(R.id.storyTextView);
mChoice1 = (Button)findViewById(R.id.choiceButton1);
mChoice2 = (Button)findViewById(R.id.choiceButton2);
loadPage();
}
private void loadPage() {
Page page = mStory.getPage(0);
Drawable drawable = getResources().getDrawable(page.getImageId());
mImageView.setImageDrawable(drawable);
String pageText = page.getText();
//Add the name if placeholder included. it wont add if no placeholder
pageText = String.format(pageText, mName);
mTextView.setText(page.getText());
mChoice1.setText(page.getChoice1().getText());
mChoice2.setText(page.getChoice2().getText());
}
public Story() { mPages = new Page[7];
mPages[0] = new Page(
R.drawable.page0,
"On your return trip from studying Saturn's rings, you hear a distress signal that seems to be coming from the surface of Mars. It's strange because there hasn't been a colony there in years. Even stranger, it's calling you by name: \"Help me, %1$s, you're my only hope.\"",
new Choice("Stop and investigate", 1),
new Choice("Continue home to Earth", 2));
6 Answers

Paul Harding
18,822 PointsSorry one slight change to my onCreate method:
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_story);
Intent intent = getIntent();
mName = intent.getStringExtra(getString(R.string.key_name));
//Log.d(TAG, mName);
if (mName == null){
mName = "Friend";
}
mImageView = (ImageView) findViewById(R.id.storyImageView);
mTextView = (TextView) findViewById(R.id.storyTextView);
mChoice1 = (Button) findViewById(R.id.choiceButton1);
mChoice2 = (Button) findViewById(R.id.choiceButton2);
loadPage();
}

Andrew D.
2,937 PointsHey guys.. I had the same problem, but you simply need to delete the String declaration in 'String mName = intent.getStringExtra(getString(R.string.key_name));' and simply set mName, like so: 'mName = intent.getStringExtra(getString(R.string.key_name));'
Before Ben altered the code, we declared name as a string. But now that it's a private variable of our class and already declared as a string when we made it a private variable, we don't need to put 'String' in front, we simply set mName equal to the key_name, which is whatever the user types in.

Taif Rahim
1,337 PointsIt's true. I'm very sorry I didn't comment here on that earlier. I've also had to change something (that's automatically generated) in the storyactivity.xml. It was the:
tools:context="nightscope.com.interactivestory.ui.MainActivity"
Not sure if this has anything to do with this or my own fault though.

Martynas Kairys
4,959 PointsIt works when I type a name. But if I leave it empty then I have Text+empty(name). Shouldn't I get "Friend" if I leave the first page empty?

Vincent Rickey
5,581 PointsSo when I had this issue my fix was to change the line mTextView.setText(page.getText); to mTextView.setText(pageText);
You have applied the formatting to the "copy" string pageText with pageText = String.format(pageText, mName);, but haven't modified the string of the TextView. You called the getter of page, so instead of replacing the TextView's text with the new "copy" formatted string, you "replaced" it with what was already there.

Taif Rahim
1,337 PointsI have the exact same thing I have copied Paul Harding's code and compared to mine, aside from a few enters, no actual differences. Now the name is replaced by null. I can't figure out why.
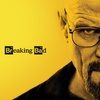
alexvalladares
21,478 PointsI guess your error is to declare twice the mName variable. At the beginning you declare it as private String mName as a method variable, and beside, in the onCreate method, you declare it again as String mName to get the intent value. You should code just
mName = intent.getStringExtra(getString(R.string.key_name));
Otherwise, method loadPage() is trying to access a variable that is not initialized (the one that is assigned with the intent value only "lives" within the onCreate() method), thus you get a null result.
Hope this helps!

Paul Harding
18,822 PointsYou missed the step where you set name to a member class variable in StoryActivity:
Add this to your class variables:
private String mName;
This is my onCreate method:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story);
Intent intent = getIntent();
String mName = intent.getStringExtra(getString(R.string.key_name));
//Log.d(TAG, mName);
if (mName == null){
mName = "Friend";
}
mImageView = (ImageView) findViewById(R.id.storyImageView);
mTextView = (TextView) findViewById(R.id.storyTextView);
mChoice1 = (Button) findViewById(R.id.choiceButton1);
mChoice2 = (Button) findViewById(R.id.choiceButton2);
loadPage();
}
Here is my loadPage Method:
private void loadPage(){
Page page = mStory.getPage(0);
Drawable drawable = getResources().getDrawable(page.getImageId());
mImageView.setImageDrawable(drawable);
String pageText = page.getText();
// Add the name if the placeholder was included //
pageText = String.format(pageText, mName);
//Log.d(TAG, mName);
mTextView.setText(pageText);
mChoice1.setText(page.getChoice1().getText());
mChoice2.setText(page.getChoice2().getText());
}

Noah Schill
10,020 PointsHello, I tried this but got the name replaced by null.
Zachary Zell
4,747 PointsZachary Zell
4,747 Pointspackage com.zachary.interactivestory.ui;
import android.content.Intent; import android.support.v7.app.ActionBarActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText;
import com.zachary.interactivestory.R;
public class MainActivity extends ActionBarActivity {
}