Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial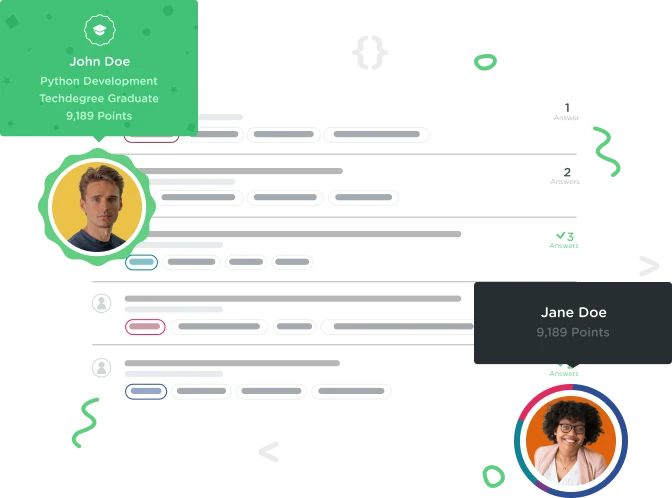
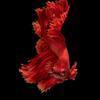
Michael Williams
Courses Plus Student 8,059 PointsWhy is it a no no to put my console.log statement inside the variable creating a new Google Maps marker?
I understand all the code (yay!), but why can't I put the console.log
statement inside the variable creating a new marker?
<!-- This is the corresponding "starter code" for 04_Hello Map in Udacity and Google's Maps
API Course, Lesson 1 -->
<html>
<head>
<!-- styles put here, but you can include a CSS file and reference it instead! -->
<style type="text/css">
html, body { height: 100%; margin: 0; padding: 0; }
#map { height: 100%; }
</style>
</head>
<body>
<!-- TODO 1: Create a place to put the map in the HTML-->
<div id="map"></div>
<script>
var map;
var markers = [];
function initMap() {
// Constructor creates a new map - only center and zoom are required.
map = new google.maps.Map(document.getElementById('map'), {
center: {lat: 40.7413549, lng: -73.9980244},
zoom: 13
});
var locations = [
{title: 'Park Ave Penthouse', location: {lat: 40.7713024, lng: -73.9632393}},
{title: 'Chelsea Loft', location: {lat: 40.7444883, lng: -73.9949465}},
{title: 'Union Square Open Floor Plan', location: {lat: 40.7347062, lng: -73.9895759}},
{title: 'East Village Hip Studio', location: {lat: 40.7281777, lng: -73.984377}},
{title: 'TriBeCa Artsy Bachelor Pad', location: {lat: 40.7195264, lng: -74.0089934}},
{title: 'Chinatown Homey Space', location: {lat: 40.7180628, lng: -73.9961237}}
];
for (var i = 0; i < locations.length; i++) {
var position = locations[i].location;
var title = locations[i].title;
var marker = new google.maps.Marker({
map: map,
position: position,
title: title,
animation: google.maps.Animation.DROP,
id: i
console.log("I just did some shit, successfully!"); // <-------- Why is this a no no?
});
}
}
</script>
<script async defer
src="https://maps.googleapis.com/maps/api/js?key=DONT_STEAL_MY_KEY_PLZ&v=3&callback=initMap">
</script>
<script>
console.log("I just did a map!");
</script>
</body>
</html>
2 Answers
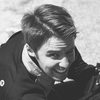
Brandon Leichty
Full Stack JavaScript Techdegree Graduate 35,193 PointsHey Michael,
Nice work so far!
The reason you’re getting an error is because you’re actually passing in an object to google.maps.Marke(). And since your you’re working with an object, you must use key value pairs.
Such as:
{
key: value,
logMessage: function () {
console.log(“I created something cool!”);
}
}
Does that make sense? If not let me know! (I’m typing this from my phone).
I’d suggest placing your console log somewhere else in your code (probably at the end of your init() function), as the Google Maps API won’t do anything with the console log inside the object.
Hope that helps! -Brandon
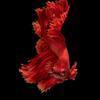
Michael Williams
Courses Plus Student 8,059 PointsAh, yes. That makes complete sense. Also explains why placing it within the loop proper it works. Thank you, Brandon.