Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial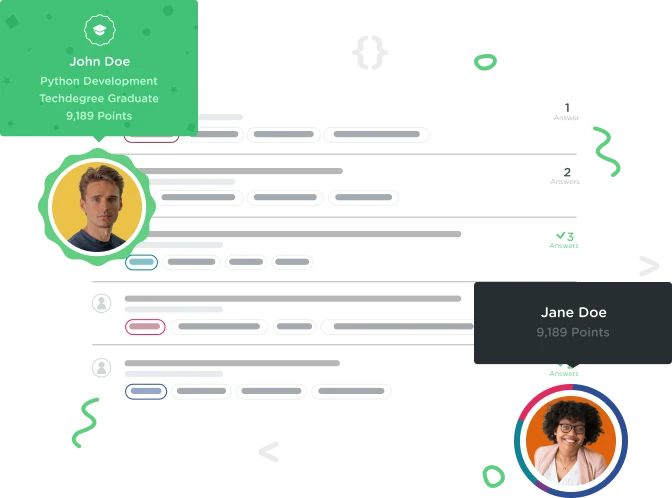

Lewis Cowles
74,902 PointsWhy is it confusing to create an Instance of User to create a User?
You stated its confusing to create an instance of User model to create new users, but is it not more error-prone and potentially troublesome to not create a null or default User instance to create a user instance?
1 Answer
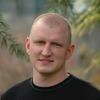
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsSorry your question went so long without an answer. If you are still curious why.
Instance Method
Can only be called if an Object has been instantiated. Uses 'self' as first parameter.
Class Method
Can be called WITHOUT instantiating an Object. Uses 'cls' as first parameter
Lets try and MAKE IT WORK, I'll throw in some comments
# Simplified User Class without models
class User:
def __init__(self, username=None):
self.username = username
def create_inst_user1(self):
# returns obj instance of itself
return self
def create_inst_user2(self, username):
# returns instance created, using name given.
return User(username)
@classmethod
def create_class_user(cls, username):
# returns the instance it created from name given.
return cls(username)
user_1 = User() # instantiated, no username defaults to None
print('\nuser_1: {}\nObj: {}\n'.format(user_1.username, user_1))
user_2 = User('Me')# instantiated with Username
print('\nuser_2: {}\nObj: {}\n'.format(user_2.username, user_2))
user_3 = User.create_class_user('Another Me') # works, with instantiating
print('\nuser_3: {}\nObj: {}\n'.format(user_3.username, user_3))
# user_4 = User.create_instance_user()
# wont work, calling instance method from Class
# Lets try to MAKE it work though
# user_2 is instantiated from User Class so maybe we can....
user_4 = user_2.create_inst_user1()
# Basically returned user_2's name, always will.
print('\nuser_4: {}\nObj: {}\n'.format(user_4.username, user_4))
user_5 = user_2.create_inst_user2('Another Instance Me')
# This technically worked, but we had to instantiated a completely different
# object just so we could create an object with a different name? Ew.
print('\nuser_5: {}\nObj: {}\n'.format(user_5.username, user_5))
# ---- OUTPUT ----
# user_1: None
# Obj: <__main__.User object at 0x000002A206746DA0>
# user_2: Me
# Obj: <__main__.User object at 0x000002A206746EB8>
# user_3: Another Me
# Obj: <__main__.User object at 0x000002A206746EF0>
# user_4: Me
# Obj: <__main__.User object at 0x000002A206746EB8>
# user_5: Another Instance Me
# Obj: <__main__.User object at 0x000002A206746E80>
Take note especially of user_2 and user_4 memory reference. They are the exactly the same. user_4 IS user_2 uh oh.
Does this help?