Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial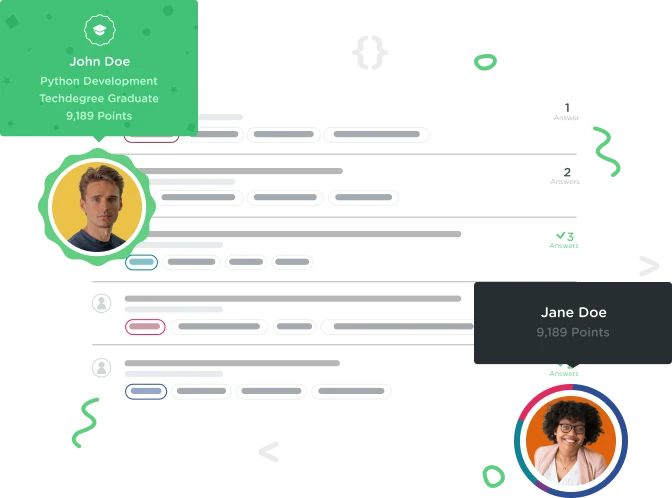

Alfredo Prince
6,175 PointsWhy is it giving me this error?
The error: Make sure you are using 'prepare' instead of 'query' and binding your parameter before execution. Why? isn't that what i did?
<?php
function get_member($member_id) {
include("connection.php");
try {
$results = $db->prepare(
"SELECT member_id, email, fullname, level
FROM members
WHERE member_id = $member_id"
);
$results->bindParam($member_id);
$results->execute();
} catch (Exception $e) {
echo "bad query";
}
$members = $results->fetchAll();
return $members;
}
1 Answer
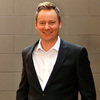
Simon Woodard
6,545 PointsHi Alfredo, you are indeed using a prepare statement but you're not binding the parameter correctly.
You first need to remember that parameters in a prepared statement need to be identified with a '?'. Secondly because multiple '?' parameters can be used in a prepared statement you also need to target which '?' you want to bind a variable too.
This means your prepare statement becomes:
$results = $db->prepare(
"SELECT member_id, email, fullname, level
FROM members
WHERE member_id = ?"
);
and your bind statement becomes:
$results->bindParam(1,$member_id)
Notice we've used the '?' in the prepare statement, then in our bindParam statement we say we want to bind the $member_id variable to the first '?'
Riku S.
11,322 PointsRiku S.
11,322 PointsHi Alfredo, try to change $member_id inside your database query to quistion mark. So it will be ...WHERE member_id = ?