Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial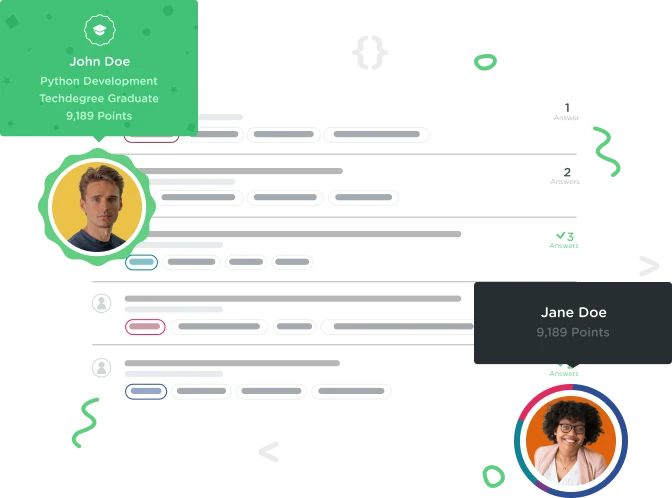

Ian Hilton
6,223 PointsWhy is it necessary to evaluate a condition inside the "do" portion of the loop, isn't that "while's" job?
I have a fundamental misunderstanding of this exercise. Why doesn't the code I wrote below work? I have given the computer something to do, while guess and randomNumber do not match.
var randomNumber = getRandomNumber(10);
var guess;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
do {
guess = prompt('Guess a number between 1 & 10');
} while (guess !== randomNumber)
document.write('you guessed correctly')
In the exercise, the condition is evaluated inside the loop and then assigned to a variable and then the variable is tested in the (while). Why would you evaluate a condition inside the do loop when that is the job of the (while)? I'm most grateful for any insight, I'm completely lost at this point...
Below is the code the exercise suggests...
var randomNumber = getRandomNumber(10);
var guess;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
do {
guess = prompt('I am thinking of a number between 1 and 10. What is it?');
if (parseInt(guess) === randomNumber) {
correctGuess = true}
} while (! correctGuess)
document.write('<h1>You guessed the number!</h1>')
2 Answers
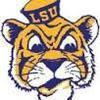
Cena Mayo
55,236 PointsHi Ian,
As a preface, MDN's explanation on do-while loops is really good, although it takes a minute to process it.
Here's what it says in a nutshell:
The do will run at least once, and then continue to run as long the condition stated in the while block evaluates to TRUE. Once this condition evaluates to FALSE, the code passes on to the next statement after the do-while loop.
Let's look at the preferred code you provided above:
var randomNumber = getRandomNumber(10);
var guess;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
do {
guess = prompt('I am thinking of a number between 1 and 10. What is it?');
if (parseInt(guess) === randomNumber) {
correctGuess = true}
} while (! correctGuess)
document.write('You guessed the number!')
Do runs once, printing the prompt. It then takes in the 'guess' (passing it through the parseInt method to ensure that it's a number, not a string). Then it asks: 'is 'guess' the same number as 'randomNumber'? If so, change 'correctGuess' to true. Check the while condition. In the case of a correct guess, (! correctGuess) evaluates to FALSE. Why? Because correctGuess at this point is TRUE, and NOT TRUE (! correctGuess) = FALSE. Since the while condition is now false, we exit the loop and print out the winning statement.
When you put in an incorrect guess, the while loop evaluates to TRUE (because correctGuess is still false, and NOT FALSE = true). Since the do loop will continue to run as long as the condition in the while loop is true, it will continue to print out the prompt until a correct guess is entered, and end, as discussed in the first paragraph.
Does that help?
Best, Cena

Max Gallant
15,500 PointsNot sure if this is still active, but the reason your code wasn't working is that you don't parse the users input as an integer. The !== comparator compares value and data type and you were comparing a string to an integer, which will never result in true.
Ian Hilton
6,223 PointsIan Hilton
6,223 PointsFirst let me thank you for the explanation and the link!
So if I understand correctly, in order for a do {}while() to work you have to monkey around with the variables inside the do portion to create a true or false condition for the while() to evaluate? You can't just use a (this !=== that) as the condition to be met?
I value your time and thank you for it.
Best,
Ian