Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial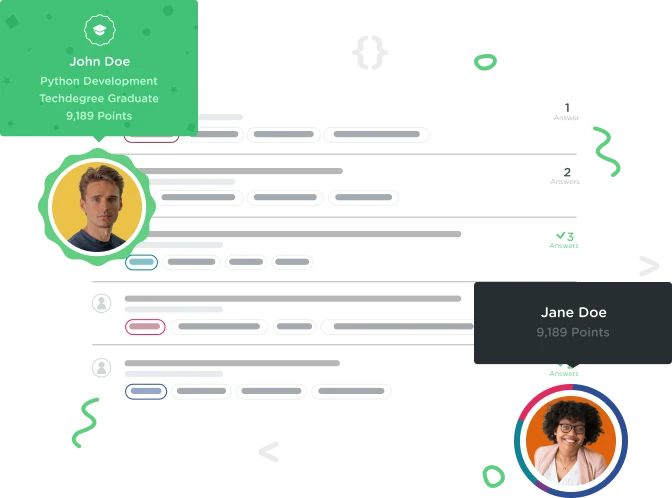

nikhil davasam
2,182 Pointswhy is it not executing
even though i close the braces properly it is not executing showing a error message - reached end of file while parsing
class Example {
public static void main(String[] args) {
GoKart kart = new GoKart("purple");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
try {
kart.drive(42);
} catch(IllegalArgumentException iae) {
System.out.println( iae );
}
}
class GoKart {
public static final int MAX_BARS = 8;
private int barCount;
private String color;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
if (laps > barCount) {
throw new IllegalArgumentException("Not enough battery remains");
}
lapsDriven += laps;
barCount -= laps;
}
}
3 Answers
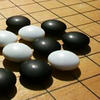
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsYes, now all your curly braces are closed, but you removed the try / catch block. You need it, your drive method still throws an IllegalArgumentException.
class Example {
int barCount;
int lapsDriven;
public static void main(String[] args) {
GoKart kart = new GoKart("purple");
if (kart.isBatteryEmpty()) {
System.out.println("The battery is empty");
}
try {
kart.drive(42);
} catch (IllegalArgumentException iae) {
System.out.println(iae);
}
}
public void drive() {
drive(1);
}
public void drive(int laps) {
if (laps > barCount) {
throw new IllegalArgumentException("Not enough battery remains");
}
}
}
I only corrected the "try" problem. In the code above there is still a logic issue. You probably shouldn't drive if your battery is empty, right? Right now you check if the battery is empty, and if yes you print a message, but try to drive anyway.
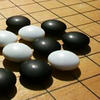
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsCount again. You're missing two closing curly braces in the Example class. Where does your if block end? Where does your main method end?

nikhil davasam
2,182 Pointsclass Example { int barCount; int lapsDriven; public static void main(String[] args) { GoKart kart = new GoKart("purple"); if (kart.isBatteryEmpty()) { System.out.println("The battery is empty"); } kart.drive(42); }
public void drive() { drive(1); }
public void drive(int laps) { if (laps > barCount) { throw new IllegalArgumentException("Not enough battery remains"); } } }
now there is another problem! it says place try methord

nikhil davasam
2,182 Pointsthanks man that was a great answer. as you are a pro developer i would like to ask you one thing even though i am knowing the concepts pretty well,i am not getting the confidence to write a seperate code for a application.and i make many errors. is there any way i can sort this out??
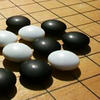
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsLook at this course from the university of Helsinki, it has a lot of very valuable exercises you can do. http://mooc.fi/courses/2013/programming-part-1/ http://mooc.fi/courses/2013/programming-part-2/ You can just jump over the course if you already know the concepts, and just do the exercises. The first ones are easy but it gets more complicated over time. You'll get in plenty of practice with different kinds of programs, and see plenty of examples of how to do a simple program that works. They have automatic testing like Treehouse (although it works a bit differently), and also have an IIRC channel to ask questions if you need help. Be patient, take it one bit at a time, and code every day.
nikhil davasam
2,182 Pointsnikhil davasam
2,182 Pointsthis actually worked but i dont understand why should we use the try and catch methord and the throw argument?
Livia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsLivia Galeazzi
Java Web Development Techdegree Graduate 21,083 PointsWell, if you try to drive but don't have any battery, things will go wrong, right? Here you'll try to remove 1 from barCount for each lap. But if you do that when barCount is already zero, you'll end up with a negative barCount. Negative battery level doesn't make sense, so if you let that happen something is going wrong! You don't want to let things happen that go against the logic of your program.
So the drive method makes sure to check there's enough battery before driving. If not, it throws an Exception, which is nothing more than a warning that something is wrong. So what happens when an exception is thrown? Well, that's what you do with the try / catch block. You decide what happens if an error happens because there's not enough battery (that's what is in the catch block). In this case you just print the error message in the console, so the user knows why things aren't working and no driving is happening.