Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial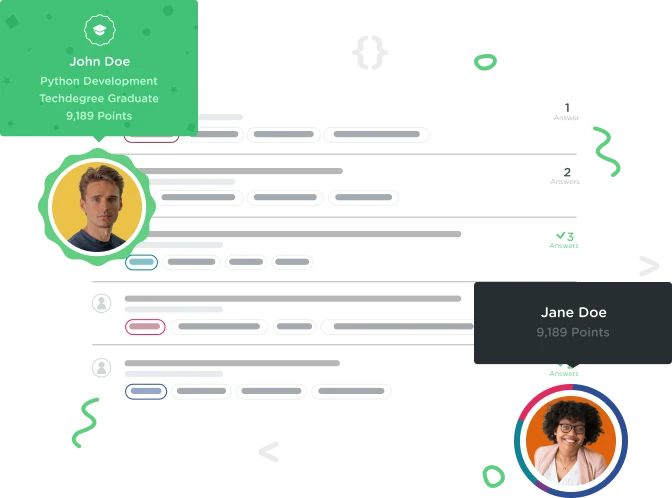
Miguel Nunez
3,266 PointsWhy is it so hard for people to explain what a return means in php in simple terms?
98 percent of every one are having troubles explaining it in simple terms a return is blah blah so basically Values are returned. Values are returned ? What the heck does that mean "Values are returned" did the php code return borrowed some values from another code and did something to it and wants to give it back it makes it seems like it's metaphorically speaking john borrowed toms ball two days later he gave tom his ball back? what is the return code returning and why did it borrowed what it borrowed and what did it borrowed and from what code did it borrowed it from I'm getting confused with the grammar return as in borrowing something and returning it and return in the coding world please clear this up with short and simple answer even if you have to use a metaphor and why would I want to use a return and for what reason?
3 Answers
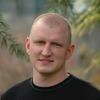
Chris Howell
Python Web Development Techdegree Graduate 49,610 PointsThink of a function as a "magic box" that does something for you when you "call upon it" by its name. Generally you would want to create a function to do a single task, this helps keep your code more flexible when you need to make changes.
Now this magic box could potentially do anything.
- it could require that YOU must give it some input/arguments/parameters, these all are basically the same thing.
- it could require no input from you, but generate something for you and give it back to you or return something.
- it could take no input, it could return nothing back, and only print/echo something out.
Take for example this function. It has been awhile since I have done PHP but lets see if I can write some still haha.
<?php
function add_two($num){
$new_num = $num + 2; // This is a value
return $new_num; // we are returning that value
}
?>
It is a simple function, its 1 and only job is to accept 1 input from YOU when you call it by its name. This "magic box's" name is add_two. So if we call it, it will want us to give it input which it will store into a variable called $num
Now whatever $num contains (hopefully an integer data type), it will do the calculation by adding 2 to whatever is contained in $num. Once that calculation has completed it is going to return THAT calculated value back to whatever called it.
So pretend I wrote that function and its part of some PHP script and later on I need to use that to ADD TWO to every item in an array of all these numbers I have.
<?php
// here is our function again...for reference
function add_two($num){
$new_num = $num + 2; // This is a value
return $new_num; // we are returning that value
}
// pretend this list came from a database or a file.
$numbers_list = array(2,6,10,14);
foreach($numbers_list as $number):
/*
Inside this block, we are looping over every item
in the $numbers_list. So first time around is 2 then 6 then 10..etc.
This could basically go on for hundreds, thoundsands or millions of times.
Each time we do 1 iteration over this array, we are "calling upon our magic box named add_two.
*NOTE 1*: We have 4 numbers in our array, so we will do 4 iterations.
And each time we call add_two, it performs a calculation, stores the value, then returns THAT value.
*NOTE 2*: add_two doesnt really have a clue what its going to receive for input until its called.
It just knows that whatever it does get, it will store it in a temporary variable called $num.
So first time around we give input of 2. Then 4 is the value returned
*/
echo add_two($number) . ' ';
endforeach;
/*
Loop would output:
4 8 12 16
*/
?>
Now return is returning our value of 4 (if input was 2) back to where it was called from. So that line where it says echo add_two($number). add_two returned a value of 4 right back to the echo command.
I will stop there, let me know if that helps at all.
Miguel Nunez
3,266 PointsThanks Chris Howell sorry about the other message its just my head hurted a lot lol so I know now what returns are all about now example of what you said the 4 will be the return value ok it makes sense now thank you :-)
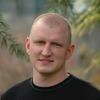
Chris Howell
Python Web Development Techdegree Graduate 49,610 PointsSo now the absolute BEST thing you can do, is go play with some code to reinforce this new understanding of return statements....until you reach another point where you get stuck again!
Best way to learn in programming is playing with code. One thing is for sure, whether you are getting errors or your code is producing wrong results or they are producing the right ones. You are learning or reinforcing what you learned. So go play with return statements! :)
Miguel Nunez
3,266 PointsThanks alot Chris :-} I have one more question man what and I know now what a return means now So I notice some examples like for example math situations will tend to give me a situation that equals the answer so for example 1+1= 2 I notice echo and a return gives the same answer and response when I see an execution of it if they as so different what are they doing given the same results and same effects why would I bother with a return if I can just do the same thing with just use an echo all the time.
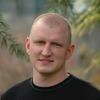
Chris Howell
Python Web Development Techdegree Graduate 49,610 PointsNo problem, it would be hard to explain these differences out. But easier to physically show you.
Are you familiar with using Team Treehouse's Workspaces?
If so I threw together a workspace that you can fork then you can Preview it in your own.
After you have forked over to your Workspace and click Preview it should open index.php up like a webpage and show you what the functions are outputting.
Be sure to check the example_functions.php file. Even add your own functions or change mine and see what happens.
Keep these things in mind:
echo statement is for outputting something.
return statement is for "handing" back something to whatever called it.
Miguel Nunez
3,266 PointsMiguel Nunez
3,266 PointsWow its making more sense now so if I did some math in a php function and I wanted the answer to be revealed to me I will have to use the return code to get my answer? Like for example 1+2 and I want my answer then I will use the return code to give me this right = 3 ? I know that's not php example but i'm trying to make that math example easy for me to understand what your really trying to say in easier terms for me to understand.
Chris Howell
Python Web Development Techdegree Graduate 49,610 PointsChris Howell
Python Web Development Techdegree Graduate 49,610 Pointsa function can be used to do almost anything, doing a Math calculation on something is just 1 thing it can do. Simple math problems are easier to understand for examples though.
For example you could make a function add two numbers (YOU GIVE IT) together and divide by two then RETURN you that answer (or value)
like this:
Miguel Nunez
3,266 PointsMiguel Nunez
3,266 PointsSorry if this sounds rude but can you just give me a straight answer to what I said about my math statement I said about if the return value will be 3? I just tend to get confused when people respond with a very long answer rather than a direct short answer.
Chris Howell
Python Web Development Techdegree Graduate 49,610 PointsChris Howell
Python Web Development Techdegree Graduate 49,610 PointsYes if you write a function that does a math calculation like you said: 1+3. if at the end of your function if you return 1+3;
That function will "give back" that answer. Which will be 4. But it hands it back to the line that originally called it, so if you set a variable equal to a function. That variable will equal whatever is returned by that function. :-)
Kevin Gates
15,052 PointsKevin Gates
15,052 PointsThis should be marked as "Best Answer".