Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial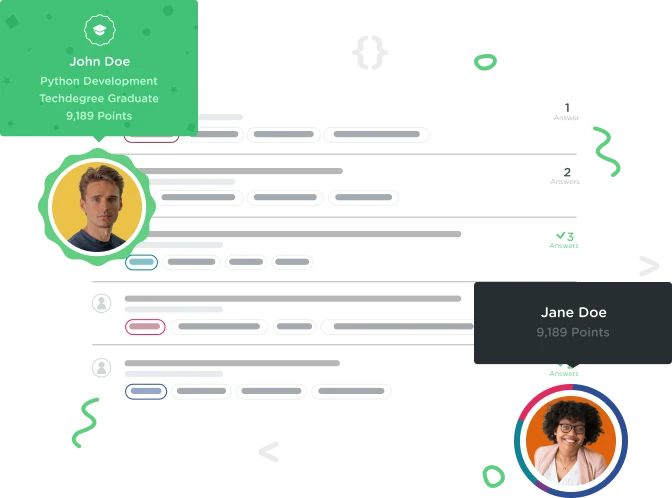

annecalija
9,031 PointsWhy is it that reuse of varible in re-instantiation of a class does not reset its attributes? what's wrong with my code?
I tried to add the suggested functionalities in the Timed Quiz App. One is letting users choose number of questions and max number of digit to be included in the question.
I'm not sure if in the code or in execution. So here's the code:
class Quiz:
questions = []
answers = []
total_correct = 0
time_elapsed = 0
def __init__(self, question_count=10, max_num=10):
self.quesitons = []
print("in QUIZ.PY q_count:{}, max_num:{}".format(question_count, max_num))
print(len(self.questions))
operations = (Add, Multiply, Subtract, Divide)
for i in range(question_count):
self.questions.append(random.choice(operations)(random.randint(1, max_num), random.randint(1, max_num)))
Here's my execution log:
Python 3.6.2 (v3.6.2:5fd33b5, Jul 8 2017, 04:57:36) [MSC v.1900 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> from quiz import Quiz
>>> qq = Quiz(1,5)
in QUIZ.PY q_count:1, max_num:5
0
>>> qq.take_quiz()
Press <ENTER> to start the 1-quesiton math quiz...
[10:46:54:232631] Start answering now!
5 x 5: 25
[10:46:56:664502] Quiz is done!
======================================================================================
| Item | Question | Correct Answer | Your Answer | Time | Mark |
======================================================================================
| 1 | 5 x 5 | 25 | 25 | 2.43 | 1 |
======================================================================================
Quiz Score: 1/1
Total Time: 2.43 sconds
>>>
>>> qq = Quiz(2,4)
in QUIZ.PY q_count:2, max_num:4
1
>>> qq.take_quiz()
Press <ENTER> to start the 3-quesiton math quiz...
[10:54:22:592186] Start answering now!
5 x 5: 25
2 + 4: 6
2 + 4: 6
[10:54:27:607857] Quiz is done!
======================================================================================
| Item | Question | Correct Answer | Your Answer | Time | Mark |
======================================================================================
| 1 | 5 x 5 | 25 | 25 | 2.43 | 1 |
| 2 | 2 + 4 | 6 | 25 | 1.66 | 1 |
| 3 | 2 + 4 | 6 | 6 | 2.07 | 1 |
======================================================================================
Quiz Score: 3/3
Total Time: 5.02 sconds
>>>
First assignment and execution went fine.
>>>qq = Quiz(1,5)
>>> qq.take_quiz()
When I reinstatiated qq=Quiz(2,4), I was expecting the questions list attribute of the class will be reset/emptied such that the new length will be 2 after _init_ is performed.
But the message is wrong, until the execution as in the complete execution log. That is also the same with the answers list attribute I have. So in the second quiz summary, evaluation is already wrong (item 2).
qq = Quiz(2,4)
...
Press <ENTER> to start the 3-quesiton math quiz...
Hoping for you enlightenment. Thanks in advance!
1 Answer

annecalija
9,031 PointsSolved by moving the attributes to _init_
class Quiz:
def __init__(self, question_count=10, max_num=10):
self.total_correct = 0
self.time_elapsed = 0
self.questions = []
self.answers = []
print("in QUIZ.PY q_count:{}, max_num:{}".format(question_count, max_num))
print(len(self.questions))
operations = (Add, Multiply, Subtract, Divide)
for i in range(question_count):
self.questions.append(random.choice(operations)(random.randint(1, max_num), random.randint(1, max_num)))
previously,
class Quiz:
questions = []
answers = []
total_correct = 0
time_elapsed = 0
def __init__(self, question_count=10, max_num=10):
print("in QUIZ.PY q_count:{}, max_num:{}".format(question_count, max_num))
print(len(self.questions))
operations = (Add, Multiply, Subtract, Divide)
for i in range(question_count):
self.questions.append(random.choice(operations)(random.randint(1, max_num), random.randint(1, max_num)))