Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial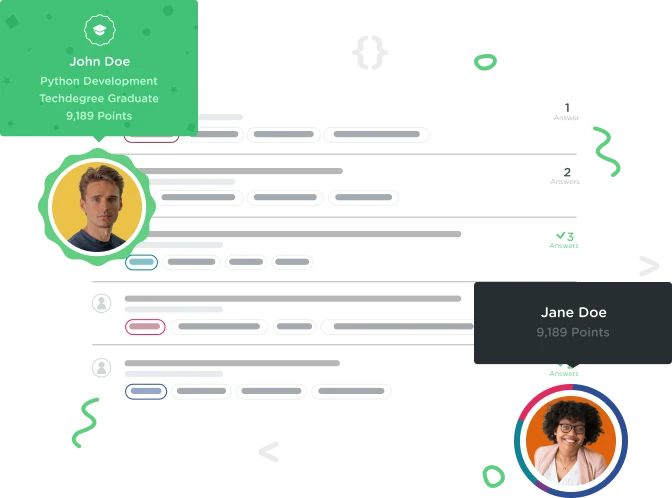

Bharat B
535 Pointswhy is list sorting dependent on output of repr
In this example, sorting the hand instance only works if the repr is fixed to return a string value?
Am not clear how sort and repr are related..
2 Answers
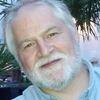
Jeff Muday
Treehouse Moderator 28,717 Points__repr()__ produces an object representation of your class. It is closely related to __str()__ which returns a string representation when either the str() or print() functions are called on your object.
__repr()__ typically returns a "structured" representation of the object like a dictionary, tuple, or a string.
If we are going to sort dice, we can use a value. But if we have compound objects that contain different types of data we would be able to use the __repr()__ to return a dictionary or tuple of the information contained in the object.
For example if we have a Student class. We would have information like name, grade, age, etc. stored as properties. Our __repr()__ representation would best return a either a tuple or a dictionary of values. This makes it easy to perform operation based on the data-- in our case, sort on one of those properties.
class Student:
def __init__(self, name, grade, age):
self.name = name
self.grade = grade
self.age = age
def __str__(self):
return self.name
def __repr__(self):
# returns a TUPLE of the properties: name, grade, age
return repr((self.name, self.grade, self.age))
Basically string representation is to visualize the salient properties. The __repr()__ allows us to easily use the properties of the object in a collection or list. Here is how to sort the list BY age
Suppose we have a list of students---
>>> student_objects = [
Student('Slim Shady','A',28),
Student('Dr. Dre','B',34),
Student('Post Malone','D',25)
]
Let's Sort
Now... we can easily sort these, but we have to use a sorting key. In this case a lambda function which returns a value we want to sort by. Don't worry if you haven't worked with "lambda" functions yet. These are pretty simple in this case the lamba REDUCES the __repr()__ to a single value to sort by, either the age, the grade, or the name.
>>> sorted(student_objects, key=lambda student: student.age) # sort by age
[('Post Malone', 'D', 25), ('Slim Shady', 'A', 28), ('Dr. Dre', 'B', 34)]
>>> sorted(student_objects, key=lambda student: student.grade) # sort by grade
[('Slim Shady', 'A', 28), ('Dr. Dre', 'B', 34), ('Post Malone', 'D', 25)]
>>> sorted(student_objects, key=lambda student: student.name) # sort by name
[('Dr. Dre', 'B', 34), ('Post Malone', 'D', 25), ('Slim Shady', 'A', 28)]
The cool thing about Python, as with most programming languages, there are always other ways to do things.
For example. If you hate using lambda functions, you could easily write your own age function.
def age(student):
return student.age
Now, lets test it to see how it works...
>>> snoop = Student('Snoop Dog','C',38)
>>> age(snoop)
38
>>> student_objects.append(snoop)
>>> student_objects
[('Slim Shady', 'A', 28), ('Dr. Dre', 'B', 34), ('Post Malone', 'D', 25), ('Snoop Dog', 'C', 38)]
>>> sorted(student_objects, key=age)
[('Post Malone', 'D', 25), ('Slim Shady', 'A', 28), ('Dr. Dre', 'B', 34), ('Snoop Dog', 'C', 38)]
>>> sorted(student_objects, key=age, reverse=True) # sort oldest to youngest
[('Snoop Dog', 'C', 38), ('Dr. Dre', 'B', 34), ('Slim Shady', 'A', 28), ('Post Malone', 'D', 25)]

Bharat B
535 PointsThank you!