Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial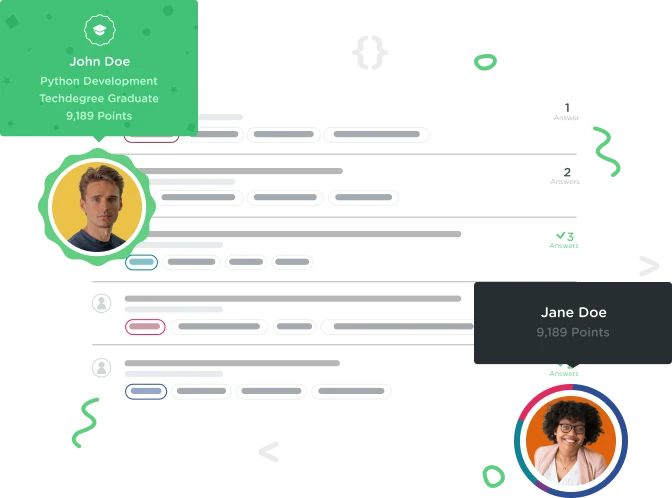
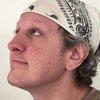
Dennis Brown
28,742 PointsWhy is MapDispatchToProps ignored here?
I certainly understand learning the various methods of dispatching methods to the redux store, and it's awesome to see bindActionCreators used this way, but I do not understand why that didn't result in using MapDispatchToProps in the end?
Like so:
const mapStateToProps = (state) => {
return {
players: state.players
};
};
const mapDispatchToProps = (dispatch) => {
return {
actions: bindActionCreators(PlayerActions, dispatch)
};
};
export default connect(mapStateToProps, mapDispatchToProps)(Application);
The actions map to this.props.actions.
4 Answers
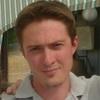
Beau Palmquist
Treehouse Guest TeacherGreat observation!
I would absolutely advocate using the mapDispatchToProps approach as it cleans up your render block and results in better performance due to the fact that the action creators are no longer created every time the container is rendered.
The intent of the original code was to show how to pass bound action creators to lower level components explicitly as named props.
Overall, I would highly encourage using the mapDispatchToProps approach for the performance and organizational gains alone.
Thanks for the question!

shubhamvinay
3,741 PointsAt 6min 13sec of video there is a line of code..const { dispatch, players } = this.props; can you please explain this line of code ?
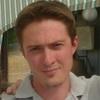
Beau Palmquist
Treehouse Guest TeacherThat particular line of code is known as Destructuring Assignment and is explained in more detail at this link:
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Destructuring_assignment
The gist of the code is that instead of writing
const dispatch = this.props.dispatch;
const players = this.props.players;
you can write
const { dispatch, players } = this.props;
and achieve the same results.

shubhamvinay
3,741 Pointsthank you for that !! I just had one more follow-up question. What is dispatch ? I understand the other elements but not really sure what "dispatch" is ??Also, how am I able to get it from props ?
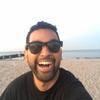
Anthony Albertorio
22,624 PointsWhat is 'dispatch'? Its mentioned so many times and yet, no one defines it.

Iting Wen
1,789 PointsI have my dispatch function like this. and it works ^_^
const mapDispatchToProps = dispatch => ({
addPlayer: (playerName) => dispatch(PlayerActionTypes.addPlayer(playerName)),
removePlayer: (index) => dispatch(PlayerActionTypes.removePlayer(index)),
updatePlayerScore: (index, score) => dispatch(PlayerActionTypes.updatePlayerScore(index, score))
});
export default connect(mapStateToProps, mapDispatchToProps)(Scoreboard);
Andrei Olar
20,827 PointsAndrei Olar
20,827 PointsI currently have my mapDispatchToProps function like this:
This makes me able to use them like this:
<AddPlayerForm addPlayer={this.props.addPlayerAction}/>
How do I use the actions individually if I will replace my mapDispatchToProps function with the one mentioned above where the returned object only has one attribute: actions ? How to I access specifically from actions? I know I can do it like this actions["addPlayer"] but I don't find it to be the right way.
Thanks.