Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial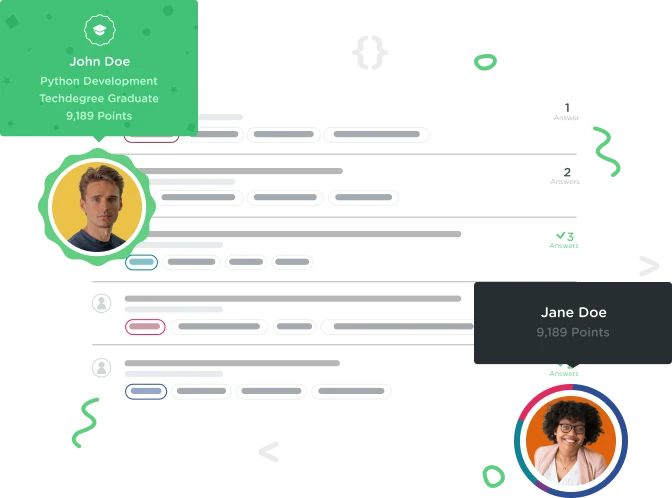
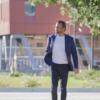
Bappy Golder
13,449 PointsWhy is my Add button not working?
I've been following along as Andrew has been showing. I've done everything as showed. But it seems my add button is still not working. Any idea what's going on here?
Here's my code.
//user interaction doesn't provide desired result.
//solution: add appropriate user interaction for achieving desired result
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button on the page
var incompleteTaskHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
var createNewTaskElement = function(taskString){
//create list item
var listItem = document.createElement("li");
//input (checkbox)
var checkBox = document.createElement("input"); //checkbox
//label
var label = document.createElement("label");
//input text
var editInput = document.createElement("input"); //text
//input:edit (button)
var editButton = document.createElement("button");
//input:delete (button)
var deleteButton = document.createElement("button");
//each element needs modifying
checkBox.type = "checkbox";
editInput.type = "text";
editButton.innerText = "Edit";
editButton.className = "edit";
deleteButton.innerText = "Delete";
deleteButton.className = "delete";
label.innerText = taskString;
//and each element needs appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//add new task
var addTask = function(){
console.log("addTask function triggerd . . .")
//create a new list item from the new task
var listItem = createNewTaskElement(taskInput.value);
//append listItem to incompleteTaskHolder
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//edit existing task
var editTsk = function(){
console.log("editTask function triggerd . . .")
//when the edit button is pressed
//if the class of the praenet is .editmode
//switch from edit mode
//label text becomes input value
//else
//switch to .editmode
//input value becomes labe's text
//toggle .editmode on the parents
}
//delete existing task
var deleteTask = function(){
console.log("deleteTask function triggerd . . .")
var listItem = this.parentNode;
var ul = listItem.parentNode;
//delete the parent list item from the ul
ul.removeChild(listItem);
}
//mark task as complete
var taskCompleted = function(){
console.log("taskCompleted function triggerd . . .")
//append the list item to #completed-tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//mark task as incomplete
var taskIncomplete = function(){
console.log("taskInComplete function triggerd . . .")
//append the list item to #incomplete-tasks
var listItem = this.parentNode;
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler){
console.log("Bind list item events");
//select taskListItem's children
var checkbox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTsk edit button
editButton.onclick = editTsk;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to the checkbox
checkbox.onchange = checkBoxEventHandler;
}
//cycle over incompleteTaskHolder ul list items
for(var i = 0; i < incompleteTaskHolder.children.length; i++){
//bind events to list items children (taskCompleted)
bindTaskEvents(incompleteTaskHolder.children[i], taskCompleted);
}
//cycle over completedTasksHolder ul list items
for(var i = 0; i < completedTasksHolder.children.length; i++){
//bind events to list items children (taskIncomplete)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
1 Answer
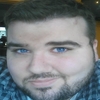
Marcus Parsons
15,719 PointsHey Bappy,
As I mentioned in your later thread, you didn't have a click event attached to your add button so it was doing nothing. You just have to put that click event somewhere on the page after the addTask
function:
addButton.onclick = addTask;