Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial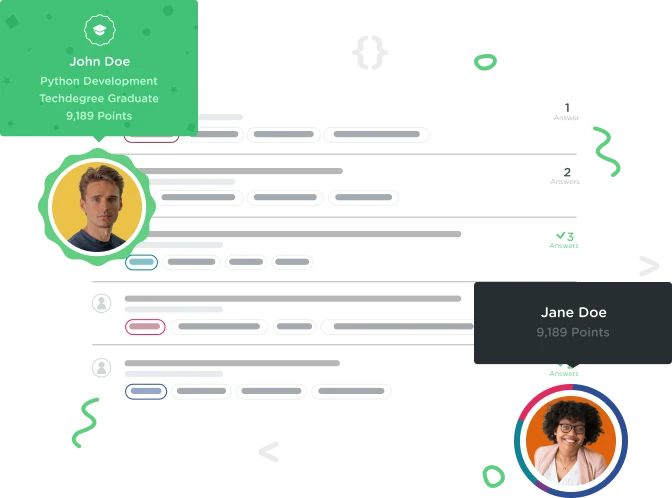

Guy Larkin
1,662 PointsWhy is my attribute assigned to the class in this code?
I was practicing with Class and init. I decided to create a Class called Child: class Child: def init(self, **kwargs): for attribute, value in kwargs.items(): setattr(self, attribute, value)
Then I created an instance: Bill = Child
Then, I decided to set some attributes: Bill.name = Bill Bill.hair = 'curly'
Then I tried to get the attribute back:
Child.hair(Bill) Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: 'str' object is not callable
But when I just call it on the Class:
Child.hair 'curly'
I'm a newb, so I can see I might have the syntax wrong the first time. But why, when I've assigned an attribute to an instance of the Child class, does calling the attribute on the class give it back?
2 Answers
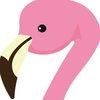
Dave StSomeWhere
19,870 PointsBecause this
Then I created an instance: Bill = Child didn't create an instance it just created reference to the same class as Child - Bill and Child are the same thing.
To create an instance you would need to include the parenthesis:
bill = Child() # create instance called bill (lower case for instance)
# use the id method to verify
# your ids will be different but Bill and Child should be the same
Bill = Child
sue = Child()
print("id of Bill is " + str(id(Bill)))
print("id of Child is " + str(id(Child)))
print("id of sue is " + str(id(sue)))
# output
id of Bill is 94898253810488
id of Child is 94898253810488
id of sue is 140248931096112

Guy Larkin
1,662 PointsThank you!