Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial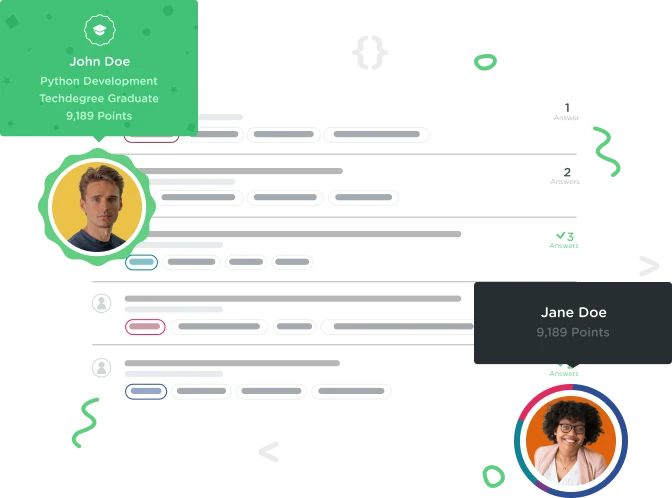

Fidel Severino
3,514 PointsWhy is my code behaving this way? I sort of passed the challenge but curious
My code prints each object five times and I cannot seem to figure out why. Can anyone explain why this is happening? Going over my code line by line it makes sense to me, but hopefully, a fresh pair of eyes will point out the issue.
I tried to go for a more modular approach in my solution without looking at Dave's yet.
// function to print to the page
function print(message) {
document.getElementById('output');
output.innerHTML = message;
}
/*
function that takes an array and goes through each item
printing the object inside
*/
function printStudentRecords(arr) {
for (var i = 0; i < arr.length; i++) {
for (var key in arr) {
html += '<h2>Student: ' + arr[i].name + '</h2>';
html += '<p>Track: ' + arr[i].track + '</p>';
html += '<p>Achievements: ' + arr[i].achievements + '</p>';
html += '<p>Points: ' + arr[i].points + '</p><hr>';
}
}
print(html);
}
var html = '';
// array of objects
var students = [
{
name: 'Joey',
track: 'iOS Developer',
achievements: 75,
points: 2000
},
{
name: 'Rassam',
track: 'Web Design',
achievements: 30,
points: 700
},
{
name: 'Melissa',
track: 'Web Development',
achievements: 78,
points: 4938
},
{
name: 'Linda',
track: 'Web Design',
achievements: 99,
points: 5984
},
{
name: 'Dave',
track: 'Android Developer',
achievements: 458,
points: 58943
}
];
printStudentRecords(students);
3 Answers
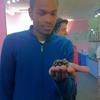
kareem Pierre
Full Stack JavaScript Techdegree Student 20,263 Pointsi have not tested it but have you tried it without the for in loop? everything else looks good to me, just keep the print inside the first loop and give that a try. i will rewrite it in a bit.

Fidel Severino
3,514 PointsThat was the issue. Just realized it after I posted the question, the for in loop wasn't really needed. Thanks for the quick response!

Ruskin Bond
1,581 Pointsone good way to understand what's happening is using the step through debugger in the chrome and add the html variable to watch list. It will be easy once you get a hang of how debugger works. No new pair of eyes will be necessary.