Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial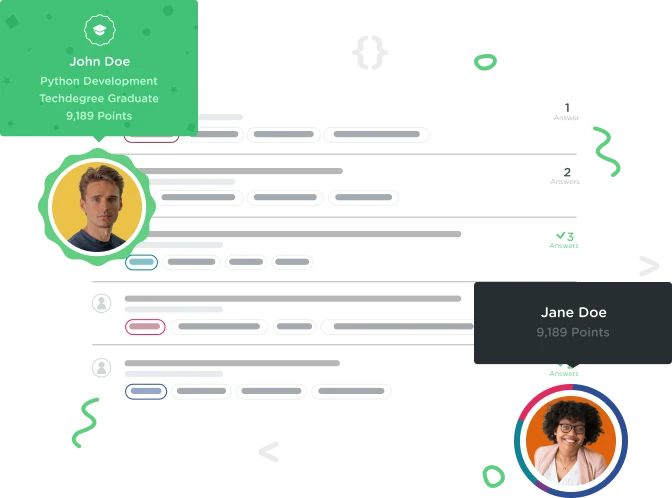

Prateek Sanyal
Courses Plus Student 2,017 PointsWhy is my code failing?
It says make sure that the initializer returns a valid instance if given a valid dictionary. There are no compilation errors and everything seems to work on xcode.
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String: String]) {
guard let bookPrice = dict["price"], let bookPubDate = dict["pubDate"], let bookAuthor = dict["author"], let bookTitle = dict["title"] else {
return nil
}
title = bookTitle
author = bookAuthor
price = bookPrice
pubDate = bookPubDate
}
}
1 Answer

Tobias Helmrich
31,602 PointsHey Prateek,
you almost got it right, good job! :) However you have one problem in your code: Note that price
and pubDate
can be nil
as they have optional types. So you shouldn't use guard
for them but just for title
and author
because right now init
also returns nil
when price
or pubDate
are nil
which shouldn't be the case. You can then assign price
and pubDate
after the else
of guard
.
Like so:
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String: String]) {
guard let bookAuthor = dict["author"], let bookTitle = dict["title"] else {
return nil
}
let bookPrice = dict["price"]
let bookPubDate = dict["pubDate"]
title = bookTitle
author = bookAuthor
price = bookPrice
pubDate = bookPubDate
}
}
I hope that helps! :)
Prateek Sanyal
Courses Plus Student 2,017 PointsPrateek Sanyal
Courses Plus Student 2,017 PointsThanks buddy! I don't know why I didn't think of this.
Tobias Helmrich
31,602 PointsTobias Helmrich
31,602 PointsNo problem, glad I could help!