Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial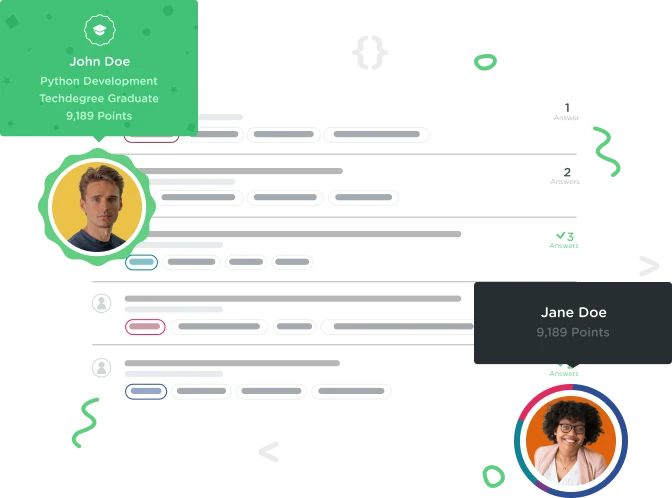
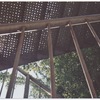
Jimmy Names
10,371 PointsWhy is my code generating 48 divs??
var html = '';
var red;
var green;
var blue;
var rgbColor;
for (var i=0; i<=10; i++) {
var red = Math.floor(Math.random() * 256 );
var green = Math.floor(Math.random() * 256 );
var blue = Math.floor(Math.random() * 256 );
var rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
document.write(html);
}
This is generating loads of div's rather than just 10.. any ideas?
Thanks
2 Answers

Tim Knight
28,888 PointsHi Jimmy,
Two things... since you're defining your variables at the top with var you don't need to use var again on the inside of your loop... it's already in the global scope.
Next, just move your document.write outside of your loop and that should solve why you're getting so many more divs than you're expecting.
var html = '',
red,
green,
blue,
rgbColor;
for (var i=0; i<=10; i++) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
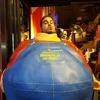
Anthony Santiago
10,082 PointsYou still have redundant code, the random number generator should be a function called for each color within the loop and the Math.random should have a (+1) after the 256. If not, you will never get the 256.

Derek Gibson
6,531 PointsWe only want values from 0 to 255, which is why we aren't using the +1 after Math.floor().
Jimmy Names
10,371 PointsJimmy Names
10,371 Pointsahh cheers man! digging how you created those variables - never seen it done like that b4
Brian Hankins
5,735 PointsBrian Hankins
5,735 PointsTim,
I'm not understanding the document.write() being outside the loop.
Can you explain why the document.write(html) must be outside of the loop to make this work? The video(s) never really explained this.
I read this loop as each time the loop runs create a random circle (css specifies these to be circles) and then print out 1 random color circle with the document.write(html).
Also, why does it print out 55 (in my case) when the document.write(html) is inside the loop?
Thanks,
Brian
Tim Knight
28,888 PointsTim Knight
28,888 PointsBrian,
It's because of how the html variable is defined. Since the html variable starts as blank, but after the first loop is never black again.
Now, the html variable is then set as itself plus the new line. So if you have the document.write inside the loop this is kind of what happens.
html
starts as blankSo in those three iterations of the loop you now have 1 + 2 + 3 (6) divs, instead of three.
By putting the write on the outside of the loop you let the
html
variable build up all of the divs in the list and then just output it once.Brian Hankins
5,735 PointsBrian Hankins
5,735 PointsTim,
Thanks!
I didn't realize that variable could append. I always thought that it would overwrite the last
Tim Knight
28,888 PointsTim Knight
28,888 PointsThat's what
+=
is doing here. You'e basically saying:html = html + newvalue
So it's "the html value is equal to its current self, plus this new string".