Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial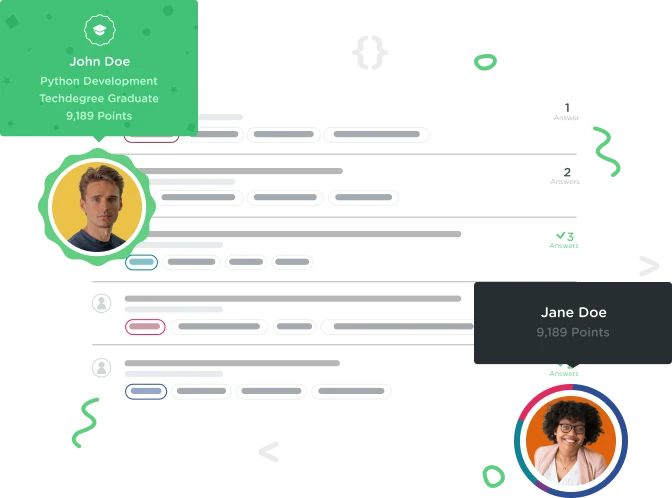

Brett Cunningham
3,251 Pointswhy is my code is producing list items that are undefined? I believe there is something wrong with my rooms[i].available
My Code Below:
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4 && xhr.status === 200) {
var rooms = JSON.parse(xhr.responseText);
var statusHTML = '<ul class="bulleted">';
for (var i=0; i<rooms.length; i += 1) {
if (rooms[i].available === true) {
statusHTML += '<li class="empty">';
} else {
statusHTML += '<li class="full">';
}
statusHTML += rooms[i].room;
statusHTML += '</li>';
}
statusHTML += '</ul>';
document.getElementById('roomList').innerHTML = statusHTML;
}
};
xhr.open('GET', '../data/rooms.json');
xhr.send();
2 Answers
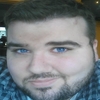
Marcus Parsons
15,719 PointsHey Brett,
First, let me ask you, is this snippet of code sitting on the same page as your code for the employees list? The code looks good.

Brett Cunningham
3,251 PointsThanks Marcus that worked! Lesson learned... the only difference is that you made new variables for the statusHTML and the xhr object. I'll keep that in mind.
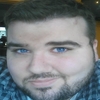
Marcus Parsons
15,719 PointsNo problem! Also, there was a change to the unordered list in the rooms part, because its class should be "rooms" not "bulleted".
You could have reused those same variables if each section of code was wrapped within a function, because functions create local variables that are destroyed upon the ending of the function so you'd be safe re-using the code like so:
function getEmployeeData() {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4 && xhr.status === 200) {
var employees = JSON.parse(xhr.responseText);
var statusHTML = '<ul class="bulleted">';
for (var i=0; i < employees.length; i += 1) {
if (employees[i].inoffice === true) {
statusHTML += '<li class="in">';
} else {
statusHTML += '<li class="out">';
}
statusHTML += employees[i].name;
statusHTML += '</li>';
}
statusHTML += '</ul>';
document.getElementById('employeeList').innerHTML = statusHTML;
}
};
xhr.open('GET', '../data/employees.json');
xhr.send();
}
function getRoomData() {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4 && xhr.status === 200) {
var rooms = JSON.parse(xhr.responseText);
var statusHTML = '<ul class="rooms">';
for (var i = 0; i < rooms.length; i += 1) {
if (rooms[i].available === true) {
statusHTML += '<li class="empty">';
} else {
statusHTML += '<li class="full">';
}
statusHTML += rooms[i].room;
statusHTML += '</li>';
}
statusHTML += '</ul>';
document.getElementById('roomList').innerHTML = statusHTML;
}
};
xhr.open('GET', '../data/rooms.json');
xhr.send();
}
//Call each function
getEmployeeData();
getRoomData();
Brett Cunningham
3,251 PointsBrett Cunningham
3,251 PointsNo, I created another js file called roomWidget.js and added another script in the html file to link to this js.. Could that be the error? It looks like it loaded except for the undefined part.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsTry renaming your variables for the room JSON data to something else because when you create variables like that, you're creating in the global context, which means that they can be accessed by other parts of your code, including other scripts on the page. When you re-use the variables with other pieces of code, it can make things wonky. Here's what I did with my rooms JavaScript (see if this helps you):