Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial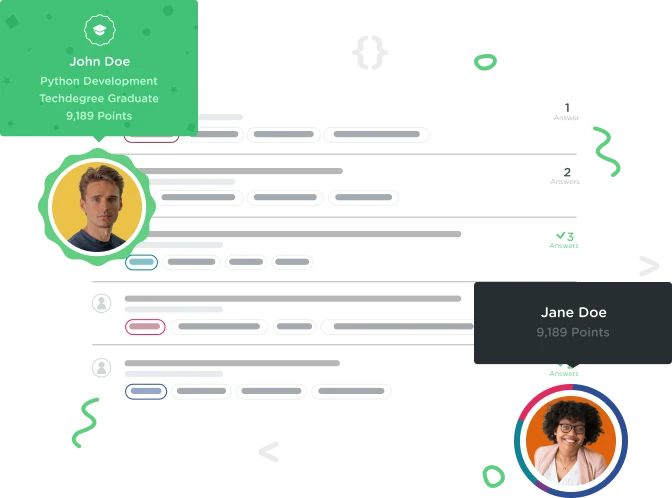
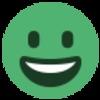
Jack Cummins
17,417 PointsWhy is my code not passing?
The instructions are: Great! Let's make one more scoring method! Create a score_yatzy method. If there are five dice with the same value, return 50. Otherwise, return 0.
class YatzyScoresheet:
def score_ones(self, hand):
return sum(hand.ones)
def _score_set(self, hand, set_size):
scores = [0]
for worth, count in hand._sets.items():
if count == set_size:
scores.append(worth*set_size)
return max(scores)
def score_one_pair(self, hand):
return self._score_set(hand, 2)
def score_chance(self, hand):
return sum(hand)
def score_yatzy(self, hand):
check_value = hand[0]
check_hand = []
for die in hand:
if die == check_value:
check_hand.append(die)
if len(check_hand) == 5:
return 50
else:
return 0
3 Answers
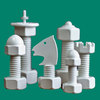
Steven Parker
231,269 PointsThis looks OK to me. So I tried pasting it directly into the challenge and it passed both tasks!
Try again?

Ross Coe
5,061 Points@gsav - that's a very neat solution, well done
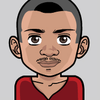
Marcos Lisboa
8,117 PointsThe question with this solution is:
What if the first element in the list is not the one repeated 5 times? Example:
[1, 4, 4, 4, 4, 4]
I think gsav solution is the best, but with a little rectification:
def score_yatzy(self, hand):
if len(set(hand)) == 1 or len(set(hand)) == 2:
return 50
else:
return 0
gsav
3,800 Pointsgsav
3,800 Pointslittle unsure why your code isn't working, but a much easier way is to convert to set and check set length.