Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial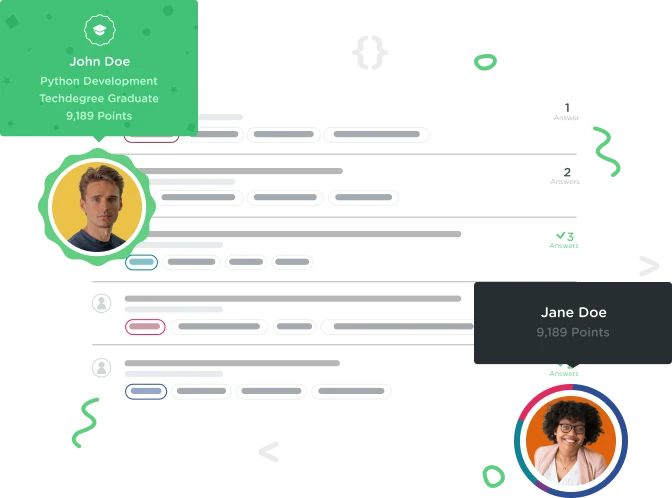

Susan Rusie
10,671 PointsWhy is my code not showing an error when I run Node with "Chalkers123"?
As far as I can see, my code looks right. However, I ran Node with both "Chalkers" and "Chalkers123" and got the same result each time. It should have run the error message when I ran Node with "Chalkers123", but it didn't. What am I missing? Here is my code:
var Profile = require("./profile.js");
var renderer = require("./renderer.js");
//Handle HTTP route GET / and POST / i.e. Home
function home(request, response){
//if url == "/" && GET
if(request.url === "/"){
//show search
response.writeHead(200, {'Content-Type': 'text/plain'});
renderer.view("header", {}, response);
renderer.view("search", {}, response);
renderer.view("footer", {}, response);
response.end();
}
//if url == "/" && POST
//redirect to /:username
}
//Handle HTTP route GET /:username i.e. /chalkers
function user(request, response){
//if url == "/...."
var username = request.url.replace("/", "");
if(username.length > 0) {
response.writeHead(200, {'Content-Type': 'text/plain'});
renderer.view("header", {}, response);
//get json from Treehouse
var studentProfile = new Profile(username);
//on "end"
studentProfile.on("end", function(profileJSON){
//show profile
//Store the values which we need
var values = {
avatarUrl: profileJSON.gravatar_url,
username: profileJSON.profile_name,
badges: profileJSON.badges.length,
javascriptPoints: profileJSON.points.JavaScript
}
//Simple response
renderer.view("profile", values, response);
renderer.view("footer", {}, response);
response.end();
});
//on "error"
studentProfile.on("error", function(error){
//show error
renderer.view("error", {errorMessage: error.message}, response);
renderer.view("search", {}, response);
renderer.view("footer", {}, response);
response.end();
});
}
}
var EventEmitter = require("events").EventEmitter;
var https = require('https');
var http = require('http');
var util = require("util");
/**
* An EventEmitter to get a Treehouse students profile.
* @param username
* @constructor
*/
function Profile(username) {
EventEmitter.call(this);
profileEmitter = this;
//Connect to the API URL (https://teamtreehouse.com/username.json)
var request = https.get("https://teamtreehouse.com/" + username + ".json", function(response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//Status Code Error
profileEmitter.emit("error", new Error("There was an error getting the profile for " + username + ". (" + http.STATUS_CODES[response.statusCode] + ")"));
}
//Read the data
response.on('data', function (chunk) {
body += chunk;
profileEmitter.emit("data", chunk);
});
response.on('end', function () {
if(response.statusCode === 200) {
try {
//Parse the data
var profile = JSON.parse(body);
profileEmitter.emit("end", profile);
} catch (error) {
profileEmitter.emit("error", error);
}
}
}).on("error", function(error){
profileEmitter.emit("error", error);
});
});
}
util.inherits( Profile, EventEmitter );
module.exports = Profile;
var fs = require("fs");
function view(templateName, values, response){
//Read from the template file
var fileContents = fs.readFileSync('./views/' + templateName + '.html');
//Insert values in to the content
//Write out the contents to the response
response.write(fileContents);
}
module.exports.view = view;
var router = require("./router.js");
//Problem: We need a simple way to look at a user's badge count and JavaScript point from a web browser
//Solution: Use Node.js to perform the profile look ups and server our template via HTTP
//Create a web server
var http = require('http');
var https = require('https');
http.createServer(function (request, response) {
router.home(request, response);
router.user(request, response);
}).listen(3000);
console.log('Server running at https://<workspace-url>/');
2 Answers
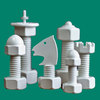
Steven Parker
229,744 PointsAre you sure you got the same result?
When the video was produced, there was no "chalkers123" account. But since then, a user named Samuel Ames signed up with "chalkers123" as his account name, probably thinking it was funny.
But he's only got a few points so the results should be a bit different. It won't cause an error anymore, though.
To see the error, try making up some really silly user name, like "bla23m77jag02". Don't use that one, though, some joker might see this posting and decide he needs it as his account name, too.

Seth Kroger
56,413 PointsSomeone created a user profile with the username "chalkers123". It's an actual user profile on the site at present, so it will come back as a valid search result.
Susan Rusie
10,671 PointsSusan Rusie
10,671 PointsYou were right. I'm glad that was what it was and not an error in my code. Thanks for your help.