Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial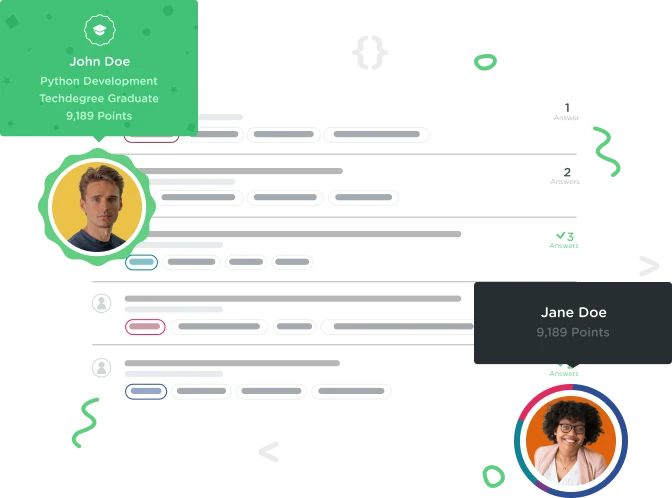

ori
2,307 PointsWhy is my code not working?
I am trying to create my own exercise based on the material taught in this chapter, but the if else statement doesn't seem to work properly. Any thoughts on what's wrong with my code? I am trying to accomplish the following items:
1) Ask user to enter his/her name 2) Determine the user's name length 3) If the user did not enter a name, show message A. If the user entered a name, show message B.
var firstName = prompt("What's your name?");
var firstNameLength = firstName.length;
var message = "There are " + firstNameLength + " characters in your name. " + firstName + "'s name is beautiful.";
console.log(firstName);
if (firstName = 'null') {
document.write("<p>You did not enter your name.</p>");
} else {
document.write("<p>" + message + "</p>");
}
3 Answers
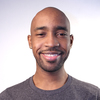
David Tonge
Courses Plus Student 45,640 PointsIt's because you're using an assignment operator instead of a equality operator.
if (firstName = 'null')
// Should be
if (firstName == 'null')

ori
2,307 PointsHi @David, thanks. I tried using == but it's still not working, see: http://jsfiddle.net/w53az0z4/

David Clausen
11,403 PointsWhat do you mean it doesn't work now? In jsfiddle you need to hit cancel when it prompt for a name. Then click run and enter name into prompt. Your javascript then prints its message. jsfiddle freezes when it process the promptbefore it loads. Hitting cancel lets jsfiddle load and then you can run it.
If you mean when you enter nothing inside the prompt that because its still getting a string just an empty string "" is not 'null'. If thats the issue then you need to catch a 0 length string
(firstName == "null" || firstName.length == 0)

ori
2,307 PointsHi David Clausen - thanks so much!
I didn't realize that if I entered nothing in the prompt and pressed okay the string becomes "" and not null. That's great. So then I see the message I was hoping to: You did not enter your name.
This does not work however when I press cancel in the prompt. I thought that if I pressed cancel, the assigned value to firstName would be null... which means that then I would still see this message: You did not enter your name.
In my Chrome Console, however it says: "Uncaught TypeError: Cannot read property 'length' of null" http://jsfiddle.net/0gdhs53p/1/
(P.S. I'm not using a script on my local machine, not the jsfiddle)

David Clausen
11,403 PointsYes cause you are mainly using jsfiddle. If the firstName was really set to null then when you call firstName.length on a null you'd get an error.
You should really set up an enviroment to work in. Have you tried bracket? or use their workspace?
Try their workspace: create a new workspace with a any envirment and blank workspace. create an index.html then create an script.js
in index.html copy this
<!DOCTYPE html>
<html lang="en">
<head>
<title>Javascript Foundation: Functions</title>
<style>
body {
background: #EEE;
font-family: sans-serif
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<script src="script.js"></script>
</body>
</html>
then in your script copy this:
var firstName = prompt("What's your name?");
console.log(firstName);
var firstNameLength = firstName.length;
var message = "There are " + firstNameLength + " characters in your name. " + firstName + "'s name is beautiful.";
if (firstName == "null") {
document.write("<p>You did not enter your name.</p>");
} else {
document.write("<p>" + message + firstname + "</p>");
}
Now if your using firefox here is the firefox console instruction https://developer.mozilla.org/en-US/docs/Tools/Browser_Console
for chrome https://developer.chrome.com/devtools/docs/console
Every time you save and click the eye in the top-right corner to preview it, just refresh that page with each save and it will update the page. The javascript will re-run and using the console instruction you can see your console.log commands.
You'll find that you cant call a length on a null, so you want to check for null right after prompt. In chrome and firefox I think cancel is null and you will get your cancel included to.
If you need more help setting this up let me know, jsfiddle is great to fiddle around real quick, but not for learning.