Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial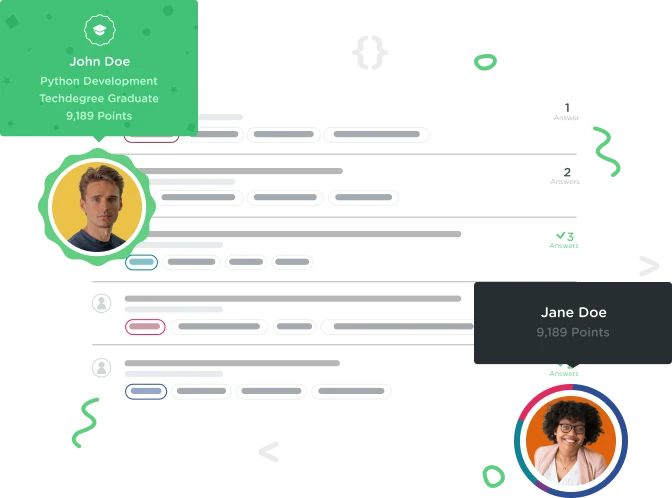

Elisabeth Hoelzl
12,206 PointsWhy is my code not working?
I wrote my code and corrected it with the video but there is no output when i run the game.
import sys
from character import Character
from monster import Dragon
from monster import Goblin
from monster import Troll
class Game:
def setup(self):
self.player = Character()
self.monsters = [
Goblin(),
Troll(),
Dragon()
]
self.monster = self.get_next_monster()
def monster_turn(self):
if self.monster.attack():
print("The {} is attacking!".format(self.monster))
want_dodge = input("Do You want to dodge?(Y/N): ").lower()
if want_dodge == 'y':
if not self.player.dodge():
self.got_hit()
print("You got hit!")
else:
print("You dodged!")
else:
print("The {} has hit you for 1 point!".format(self.monster))
self.got_hit()
else:
print("Your a lucky guy the {} isn't attacking".format(self.monster))
def player_turn(self):
player_choice = input("Do you want to attack, rest or quit?(a/r/q): ").lower()
if player_choice == 'q':
print("See you next time by.")
sys.exit()
elif player_choice == 'a':
print("You are attaking the {}".format(self.monster))
if self.player.attack():
if self.monster.dodge():
print("OH no it dodged")
else:
if self.player.leveled_up():
self.monster.hit_points -=2
else:
self.monster.hit_points -=1
print("You hit {} with your {}!".format(self.monster, self.player.weapon))
else:
print("You missed")
elif player_choice == 'r':
self.player.rest()
else:
self.player_turn()
def got_hit(self):
self.player.hit_points -=1
def get_next_monster(self):
try:
return self.monsters.pop(0)
except IndexError:
return None
def cleanup(self):
if self.monster.hit_points <=0:
self.player.experience += self.monster.experience
print("You have killed the {}!".format(self.monster))
self.monster = self.get_next_monster()
def __init__(self):
self.setup()
while self.player.hit_points and (self.monster or self.monsters):
print('\n'+'='*20)
print(self.player)
self.monster_turn()
print('-'*20)
self.player_turn()
self.cleanup()
print('\n'+'='*20)
if self.player.hit_points:
print("You win!")
sys.exit()
elif self.monsters or self.monster:
print("You lose!")
sys.exit()
Game()
1 Answer
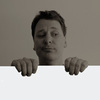
Sean T. Unwin
28,690 PointsAll the function definitions after setup()
are indented too far making them child functions of setup()
. The functions' definitions should all align with each other within class Game
.
Elisabeth Hoelzl
12,206 PointsElisabeth Hoelzl
12,206 PointsThank you next time i will look for something like this. :)