Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial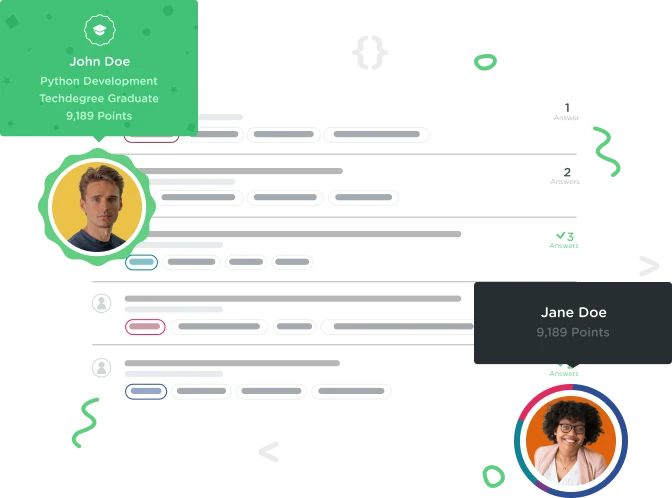

Cody Fisher
12,309 PointsWhy is my code working in my REPL, but not in this challenge?
Attached is my code. This works fine in my REPL. It does the following steps: 1) convert string to all lowercase 2) split string into a list by all spaces so the list contains all words in the string 3) create an empty dictionary to hold the word keys and their count values 4) for each word in the list - if the word is a key in the dictionary then increment its value by 1 - otherwise, the word is not yet in the dictionary so add it as a key and initialize its value to 1 5) return the object containing all of the words and their counts
Is this an issue with the way the code is being tested, or is there a bug in my code that I am not seeing?
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
string = string.lower()
word_list = string.split(" ")
word_counter = {}
for word in word_list:
if word in word_counter.keys():
word_counter[word] += 1
else:
word_counter[word] = 1
return word_counter
1 Answer
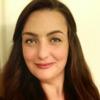
Jennifer Nordell
Treehouse TeacherHi there, Cody Fisher! There isn't a bug in the challenge, nor is there necessarily a bug in your code really. Your code will work for any data set that only contains spaces as white space, but it will fail on any code that contains new line characters or tabs as white space. The problem here is a combination of the interpretation of the instructions and your test data. The test data that was given will give you back a sort of "false positive" because it only contains spaces as white space.
This splits on spaces:
.split(" ")
But this splits on all white space (including things like tabs and new line characters):
.split()
Removing the " "
as an argument to the split method causes your code to pass with flying colors! Way to go!
Cody Fisher
12,309 PointsCody Fisher
12,309 PointsThanks! That did the trick. I also saw some people shortening my code to assigning word_list directly like this:
word_list = string.lower().split()