Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial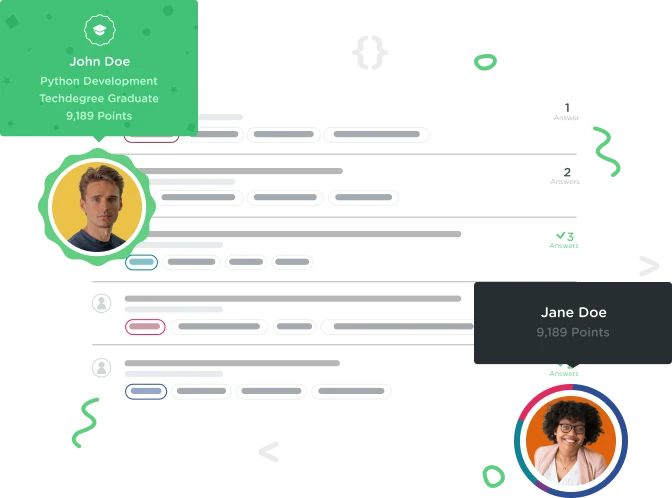

Jennifer Elliott
Front End Web Development Techdegree Student 2,183 PointsWhy is my count and random number generator off?
Please help. My code runs, but it returns the incorrect number of tries it took for the user to guess how many items were in the jar. Also, my random number generator parameter is not working correctly.
MAIN
import java.util.Scanner;
public class GuessingGame {
public static void main(String[] args) {
System.out.println("ADMINISTRATOR SETUP\n********************");
System.out.println("GUESSING GAME: Guess how many items are in the jar.\n\nSelect the type of items, and how many can reasonably fit in a jar.\nSound good? Okay let's begin!\n");
if(args.length != 2) {
Jar jar = new Jar();
jar.fillJar();
}
}
}
import java.util.Scanner;
import java.util.Random;
public class Jar {
private String itemName;
public int maxItems;
public Jar() {
Scanner scanner = new Scanner(System.in);
System.out.println("What type of item?");
this.itemName = scanner.nextLine();
System.out.println("What is the maximum amount of " + itemName + " ?");
this.maxItems = Integer.parseInt(scanner.nextLine());
System.out.println("\nPLAYER\n********************\nHow many " + itemName + " are in the jar?\nPick a number between 1 and " + maxItems);
}
public String fillJar() {
Random randomNumber = new Random();
int rand = randomNumber.nextInt(maxItems);
Scanner scanner = new Scanner(System.in);
int guess = Integer.parseInt(scanner.nextLine());
do {
Scanner scanner1 = new Scanner(System.in);
System.out.println("\nHow many " + itemName + " are in the jar? Pick a number between 1 and " + maxItems);
guess = Integer.parseInt(scanner1.nextLine());
int count = 0;
count += 1;
if(guess==rand){
System.out.println("You got it in " + count + " attempt(s)");
}
} while(guess != rand);
return "You got it in X attempt(s)";
}
}
1 Answer
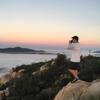
Chase Carnaroli
5,139 PointsTry putting "int count = 0;
" outside of of the do-while loop.
You have the right idea with "count += 1
", but since "int count = 0;
" is inside of the loop, each time it loops count is reset back to 0.
I think this should work.
int count = 0;
do {
Scanner scanner1 = new Scanner(System.in);
System.out.println("\nHow many " + itemName + " are in the jar? Pick a number between 1 and " + maxItems);
guess = Integer.parseInt(scanner1.nextLine());
count += 1;
if(guess==rand){
System.out.println("You got it in " + count + " attempt(s)");
}
} while(guess != rand);
Jennifer Elliott
Front End Web Development Techdegree Student 2,183 PointsJennifer Elliott
Front End Web Development Techdegree Student 2,183 PointsGood call, I didn't catch that I did that. Thanks for your help!