Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial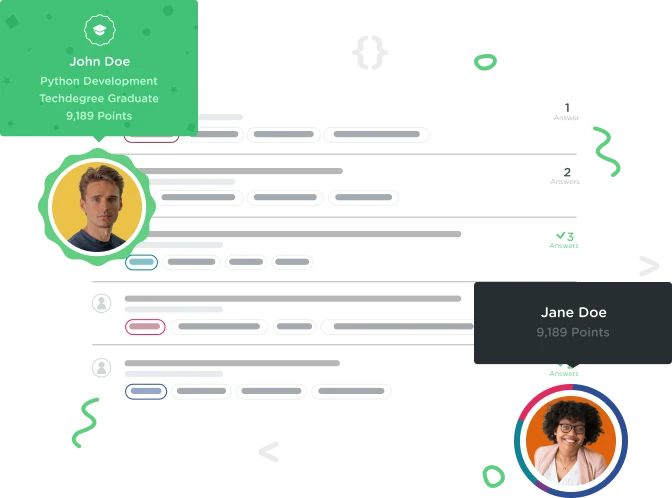

Mark Libario
9,003 PointsWhy is my javascript loop not working?
I am just trying to reenact the game of "CRAPS". A player can keep on rolling the dice as long as he doesn't roll a 7.
I wanted to make a loop that keeps on rolling 2 random die number that when combined is not equal to 7.
However, if a seven actually rolls, I want it to give an alert that "You rolled a 7, out."
function rollDieOne () {
var dieOne = Math.floor ( Math.random () * 6) + 1;
return dieOne
}
function rollDieTwo () {
var dieTwo = Math.floor ( Math.random () * 6) + 1;
return dieTwo
}
while (rollDieOne () +rollDieTwo () ==7) {
alert ("You rolled a " + (rollDieOne () + rollDieTwo ()) + ".");
}
Im doing the function right, but when i put the while function, it doesnt work anymore.
3 Answers
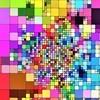
james south
Front End Web Development Techdegree Graduate 33,271 Pointsa while loop can run as few as 0 times. if the first call to the rolling functions doesn't add up to 7, the loop will not be entered. what i think you want is the loop to run while the sum is not equal to 7, in which case it should say != 7 instead of == 7. sums other than 7 would allow the loop to run, the alert would come up, and it would loop again. if the sum equals 7, the loop will exit, and afterwards you can put another alert with the message for crapping out.

Mark Libario
9,003 PointsI see. I tried a different route. But now it makes my browser lag or if its an alert, it never stops alerting even if the 7 hits.
var rollDice = rollDieOne () + rollDieTwo ()
var attempts = 0
function rollDieOne () {
var dieOne = Math.floor ( Math.random () * 6) + 1;
return dieOne
}
function rollDieTwo () {
var dieTwo = Math.floor ( Math.random () * 6) + 1;
return dieTwo
}
while ( rollDice !==7 ) {
document.write("You rolled a "+ ( rollDieOne () + rollDieTwo () ) + ".");
attempts += 1;
}
document.write ("You rolled " + attempts + " times before crapping out.");
or
var rollDice = rollDieOne () + rollDieTwo ()
var attempts = 0
function rollDieOne () {
var dieOne = Math.floor ( Math.random () * 6) + 1;
return dieOne
}
function rollDieTwo () {
var dieTwo = Math.floor ( Math.random () * 6) + 1;
return dieTwo
}
while ( rollDice !==7 ) {
document.write("You rolled a "+ rollDice + "."); /* This code just shows the same number every time idk why */
attempts += 1;
}
document.write("You rolled " + attempts + " times before crapping out.");

Mark Libario
9,003 PointsNevermind. Found it by my own!
var rollDice;
var attempts = 0
function rollDieOne () {
var dieOne = Math.floor ( Math.random () * 6) + 1;
return dieOne
}
function rollDieTwo () {
var dieTwo = Math.floor ( Math.random () * 6) + 1;
return dieTwo
}
while (rollDice != 7 ) {
var rollDice = (rollDieOne () + rollDieTwo ());
alert("You rolled a "+ rollDice + ".");
attempts += 1;
}
document.write("You rolled " + attempts + " times before crapping out.");