Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial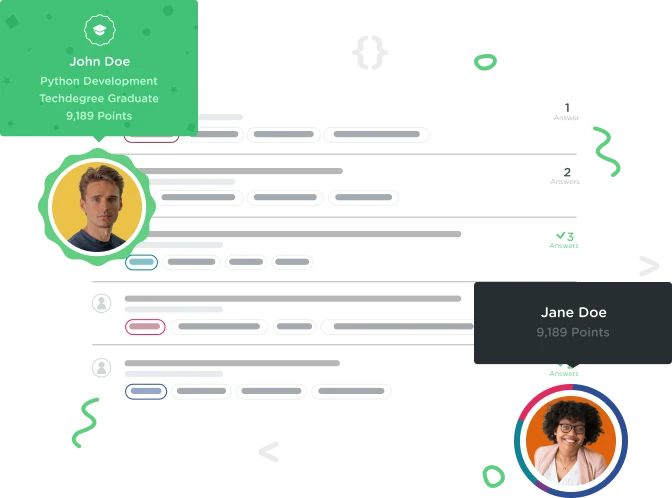
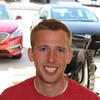
steven swensen
7,926 PointsWhy is my JavaScript not changing the 'p.descrtiption'
HTML
<!DOCTYPE html>
<html lang = "en">
<head>
<title>JavaScript Practice</title>
<meta charset = 'UTF-8'>
<link rel="stylesheet" type="text/css" href="css/style.css">
<script src="app.js"></script>
</head>
<body>
<header><h1>JavaScript Practice</h1></header>
<nav>
</nav>
<main>
<div id="pro1">
<h1>Latin Translator</h1>
<!--<button type="button" id = "dexter">Dexter</button>
<button type="button" id = "medium">Medium</button>-->
<p class="description">answer</p>
<input type="text" class="description">
<button class="description">Sinister</button>
</div>
</main>
<footer><em>Created by Steven Swensen.</em></footer>
</body>
</html>
JAVASCRIPT
const input = document.querySelector('input');
const p = document.querySelector('p.description');
const button = document.querySelector('button');
button.addEventListener('click', () => {
p.textContent = input.value + ':';
});
Moderator Edited: Markdown added so the code renders properly in the forums.
4 Answers

Erick Amezcua
21,826 PointsI tried it on a workspace and I made it work simply by changing the script position.
<!DOCTYPE html>
<html lang = "en">
<head>
<title>JavaScript Practice</title>
<meta charset = 'UTF-8'>
<link rel="stylesheet" type="text/css" href="css/style.css">
</head>
<body>
<header><h1>JavaScript Practice</h1></header>
<nav>
</nav>
<main>
<div id="pro1">
<h1>Latin Translator</h1>
<!--<button type="button" id = "dexter">Dexter</button>
<button type="button" id = "medium">Medium</button>-->
<p class="description">answer</p>
<input type="text" class="description">
<button class="description">Sinister</button>
</div>
</main>
<footer><em>Created by Steven Swensen.</em></footer>
<script src="app.js"></script>
</body>
</html>
Remember, whenever the DOM is edited, the script must be imported to the last one to make sure that the entire DOM has been loaded
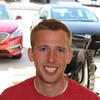
steven swensen
7,926 Pointsoh and thank you for your help. sorry where are my manners.
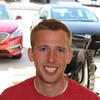
steven swensen
7,926 PointsYeah why is that? does it just have to be in the body?

Erick Amezcua
21,826 PointsIt is a good practice to put it before the body, but it can really be anywhere, as long as it is before the </ html> tag.
In your first code it does not work because it first loads the script and then loads the page content, then the script does nothing because it loads before any button exists. In any case, if you want to make sure the DOM is fully loaded you can use:
window.onload= function(){
//Your code here
};
Or with jQuery:
$('document').ready(function(){
//Your code here
});
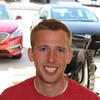
steven swensen
7,926 Pointsokay cool. I have one more question for you do you know how to section your code? for instance if i had
JavaScript
//Clickable Number Images const one = document.querySelector('input[name="one"]'); const two = document.querySelector('input[name="two"]'); const three = document.querySelector('input[name="three"]'); const four = document.querySelector('input[name="four"]'); const five = document.querySelector('input[name="five"]'); const h2Spelling = document.querySelector('h2.spelling'); one.addEventListener('click', () => { h2Spelling.textContent = 'One'; }); two.addEventListener('click', () => { h2Spelling.textContent = 'Two'; }); three.addEventListener('click', () => { h2Spelling.textContent = 'Three'; }); four.addEventListener('click', () => { h2Spelling.textContent = 'Four'; }); five.addEventListener('click', () => { h2Spelling.textContent = 'Five'; });
and I wanted to be able to collapse and open this like how you can do with a function, how would you go about doing that, so I can read the note and then click to open the section if I wanted to see or change the code. thanks again for all your help.