Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial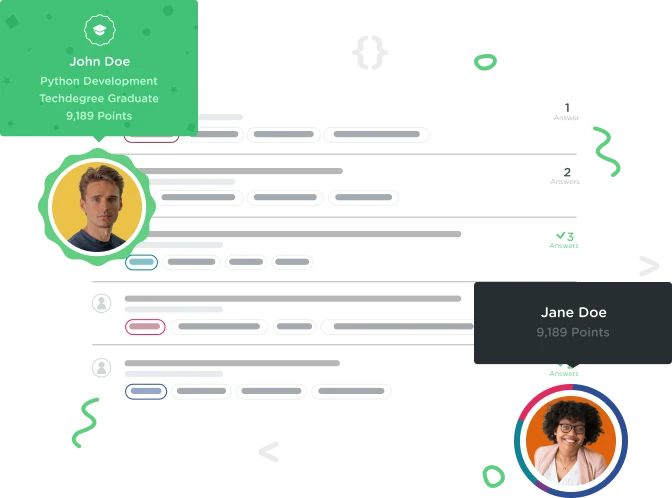
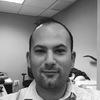
Daniel Simakovsky
Full Stack JavaScript Techdegree Student 1,996 PointsWhy is my <ol> <li> not functioning properly?
var quiz = [ ['What is the capital of New York', 'Albany'], ['What is the Capital of California', 'Sacramento'], ['What is the capital of New Jersey', 'Trenton'], ['What is the capital of Virginia', 'Richmond'] ];
var questionsRight = 0; var questionsWrong = 0; var correct = []; var wrong = [];
/* Don't NEED to declare the following right away, but it's good practice to go ahead and declare the variables you're going to use. Notice you don't need to assign any default value to them */ var question; var answer; var response; var html;
function print(message) { document.write(message); }
function buildList(arr) { var listHTML = '<ol>'; for( var i = 0; i < arr.length; i++) { listHTML = '<li>' + arr[i] + '</li>'; } listHTML += '</ol>'; return listHTML; }
for( var i = 0; i < quiz.length; i++) {
// Get the first item from array[i] and set it as question
question = quiz[i][0];
// Get the second item from array[i] and set it as answer
answer = quiz[i][1];
// Get input from the user and set it to the response variable
response = prompt(question);
if (response.toLowerCase() === answer.toLowerCase()) {
//questionsRight is initially 0. If response === answer questionsRight = 0+1
questionsRight += 1;
correct.push(question);
}
else {
//questionsWrong is initially 0. If response === answer questionsWrong = 0+1
wrong.push(question);
}
}
html = "You got " + questionsRight + " question(s) right.";
html += '<h2> You got these questions correct:</h2>';
html += buildList(correct);
html += '<h2> You got these questions wrong:</h2>';
html += buildList(wrong);
print(html);
2 Answers
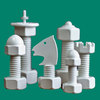
Steven Parker
229,785 PointsIt looks like you are overwriting your list here:
listHTML = '<li>' + arr[i] + '</li>';
You probably meant to append it, like this:
listHTML += '<li>' + arr[i] + '</li>'; // added "+"
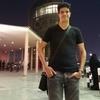
Haytham Badran
216 PointsYour issue was in this line
function buildList(arr) { var listHTML = '<ol>'; for( var i = 0; i < arr.length; i++) { listHTML = '<li>' + arr[i] + '</li>'; } listHTML += '</ol>'; return listHTML; }
you didn't make loop add each <li> created after user enter the answer and overwriting your <li>
listHTML = '<li>' + arr[i] + '</li>';
it must be
listHTML += '<li>' + arr[i] + '</li>';
to complete the ol with li elements
Follwoing is the correct code
var quiz = [ ['What is the capital of New York', 'Albany'], ['What is the Capital of California', 'Sacramento'], ['What is the capital of New Jersey', 'Trenton'], ['What is the capital of Virginia', 'Richmond'] ];
var questionsRight = 0;
var questionsWrong = 0;
var correct = []; var wrong = [];
/* Don't NEED to declare the following right away, but it's good practice to go ahead and declare the variables you're going to use. Notice you don't need to assign any default value to them */ var question; var answer; var response; var html;
function print(message) { document.write(message); }
function buildList(arr) {
var listHTML = '<ol>';
for( var i = 0; i < arr.length; i++) {
listHTML += '<li>' + arr[i] + '</li>'; // The line which is corrected
}
listHTML += '</ol>';
return listHTML;
}
for( var i = 0; i < quiz.length; i++) {
// Get the first item from array[i] and set it as question
question = quiz[i][0];
// Get the second item from array[i] and set it as answer
answer = quiz[i][1];
// Get input from the user and set it to the response variable
response = prompt(question);
if (response.toLowerCase() === answer.toLowerCase()) {
//questionsRight is initially 0. If response === answer questionsRight = 0+1
questionsRight += 1;
correct.push(question);
}
else {
//questionsWrong is initially 0. If response === answer questionsWrong = 0+1
wrong.push(question);
}
}
html = "You got " + questionsRight + " question(s) right.";
html += '<h2> You got these questions correct:</h2>';
html += buildList(correct);
html += '<h2> You got these questions wrong:</h2>';
html += buildList(wrong);
print(html);
Daniel Simakovsky
Full Stack JavaScript Techdegree Student 1,996 PointsDaniel Simakovsky
Full Stack JavaScript Techdegree Student 1,996 PointsThanks!