Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial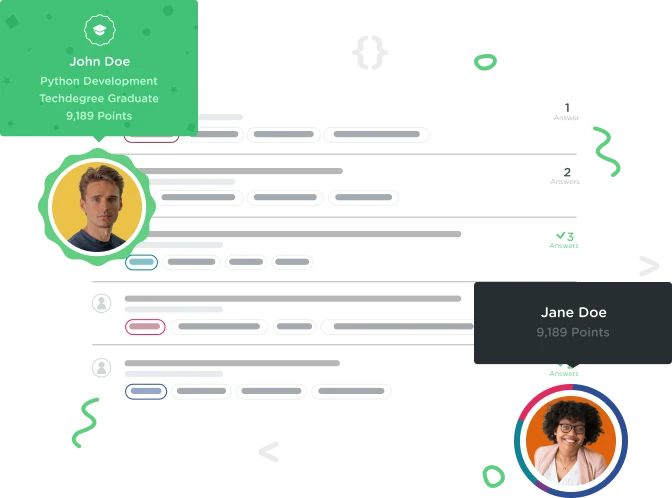

Virginia Hickman
1,862 PointsWhy is my `out of the word` code not running properly?
My code is not continuing past the first if statement checking to make sure the characters in the player's guess are in the challenge word. What gives?
import random
WORDS = (
"Treehouse",
"Python",
"Learner",
)
def prompt_for_words(challenge_word):
guesses = set()
print("What words can you find in the word {}?".format(challenge_word))
print("(Enter Q to quit)")
while True:
guess = input("{} words > .".format(len(guesses))
if guess <= challenge_word:
if len(guess) < 2:
if guess.upper() == "Q":
break
else:
print("Guesses must be at least two characters. Try again.")
continue
guesses.add(guess.lower())
else:
print("words must be in challenge word")
return guesses
def output_results(results):
for word in results:
print(" * " + word)
challenge_word = random.choice(WORDS)
player1_words = prompt_for_words(challenge_word)
player2_words = prompt_for_words(challenge_word)
print("Player 1 results: ")
player1_unique = player1_words - player2_words
print("{} guesses, {} unique".format(len(player1_words),len(player1_unique)))
output_results(player1_unique)
print("-"*10)
print("Player 2 results: ")
player2_unique = player2_words - player1_words
print("{} guesses, {} unique".format(len(player2_words),len(player2_unique)))
output_results(player2_unique)
print("Shared guesses: ")
shared_words = player1_words & player2_words
output_results(shared_words)
5 Answers
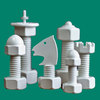
Steven Parker
231,275 PointsThis test probably doesn't do what you are expecting:
if guess <= challenge_word:
What it actually will do is determine if the guess comes before the challenge word in alphabetic order.
You may also want to revise the testing order to check for a "Q" first.

Virginia Hickman
1,862 PointsSteven Parker , I fixed the parentheses, but my code still isn't running past line 14. It keeps saying that the input of the guess isn't defined.
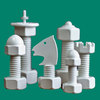
Steven Parker
231,275 PointsDid you save the changes (with Ctrl-S or the menu) before running again? I had no errors after making that change:
guess = input("{} words > .".format(len(guesses)) # I changed this line
guess = input("{} words > .".format(len(guesses))) # <-- to this

Virginia Hickman
1,862 PointsSteven Parker , I did. It actually runs fine in the workspace now, but doesn't run in my Visual Studio Code. hrm.... Thank you!

Virginia Hickman
1,862 PointsThank you Steven Parker . I realized i didn't have the latest version of python on Visual Studio Code so that's why it wasn't working on there
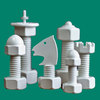
Steven Parker
231,275 PointsI'm surprised that the version would matter, since none of this code is "cutting edge" stuff. But I'm glad you were able to resolve it!

Virginia Hickman
1,862 PointsSteven Parker , the older version really didn't like the "end" parameter in the print statement.
Virginia Hickman
1,862 PointsVirginia Hickman
1,862 PointsI just decided to take out the if statements for now and just work on the core but I still can't get it to work. It keeps giving me an error when I try and add the guess input to the guesses set.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsI missed it the first time, but line 14 (the one with "input") has unbalanced parentheses. It needs one more closing parenthesis at the end.