Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial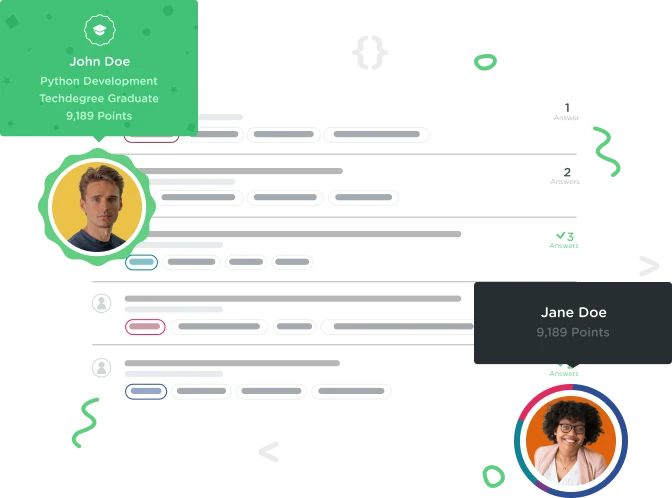
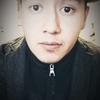
Nelson J
7,411 PointsWhy is my program not continuing to prompt user when the search doesn't match the student?
When I search the correct name and it matches the program runs great but when the search doesn't match, the program simply ends. Also, I know I am not taking care of case sensitivity in my program at this point, i am mostly worried about the logic of the bug. I can fix case sensitivity easily. Can you explain why the explained problem above continues to happen. Thank you.
var message = '';
var i;
var search = '';
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
do {
search = prompt("Enter name");
for(i = 0; search !== students[i]; i++) {
if(students[i].name === search) {
message += '<h2> Student: ' + students[i].name + '</h2>' + '<br>';
message += '<p> Track: ' + students[i].track + '</p>' + '<br>';
message += '<p> Points: ' + students[i].achievements + '</p>' + '<br>';
message += '<p> Achievements: ' + students[i].points + '</p>' + '<br>';
print(message);
}
}
} while(search !== students[i])
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
2 Answers
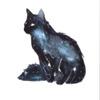
Antony .
2,824 PointsHi Nelson J
There's a few reasons why the code inside the do-while loop isn't working.
-
I can see that you declared the variable i at the top of your code, but the variable i inside the conditional of the for-loop does not work in the way you expect. The i variable does not start to increment unless it's inside the curly braces of the for-loop. Therefore the code underneath wouldn't work. The for-loop of course still runs, but only once, after it sees that the condition inside it is false it exits the loop. The reason
search !== students[i]
is false is because the i variable does not start to increment unless, like i said before, it's inside the curly braces.for(i = 0; search !== students[i]; i++)
Now the reason prompt isn't showing more than once is because the condition inside the while loop is false. How? Because your while loop does not have access to your incrementing/iterating i that of course ONLY belongs inside the for-loop.
while(search !== students[i])
So what i did is change both of those issues to something that would work.
for(i = 0; i < students.length; i++)
var bool = false;
do {
search = prompt("Enter name");
for(i = 0; i < students.length; i++) {
console.log(i)
if(students[i].name === search) {
message += '<h2> Student: ' + students[i].name + '</h2>' + '<br>';
message += '<p> Track: ' + students[i].track + '</p>' + '<br>';
message += '<p> Points: ' + students[i].achievements + '</p>' + '<br>';
message += '<p> Achievements: ' + students[i].points + '</p>' + '<br>';
print(message);
bool = true;
}
}
} while(!bool)
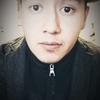
Nelson J
7,411 PointsI did the following, and it works but now, the else statement won't execute at all, it just ends the loop when a string that does not match any of the other conditions is entered.
do {
search = prompt("Enter name");
search.toUpperCase();
for(i = 0; search !== students[i].name; i++) {
students[i].name.toUpperCase();
}
if(search === students[i].name) {
message += '<h2> Student: ' + students[i].name + '</h2>' + '<br>';
message += '<p> Track: ' + students[i].track + '</p>' + '<br>';
message += '<p> Points: ' + students[i].achievements + '</p>' + '<br>';
message += '<p> Achievements: ' + students[i].points + '</p>' + '<br>';
print(message);
}
else if(seach === 'Quit') {
break;
}
else {
search = prompt('Enter name');
}
} while(search !== students[i].name);
Your solution works. But i don't why my doesn't now.
Nelson J
7,411 PointsNelson J
7,411 PointsHI @antonypesantez