Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial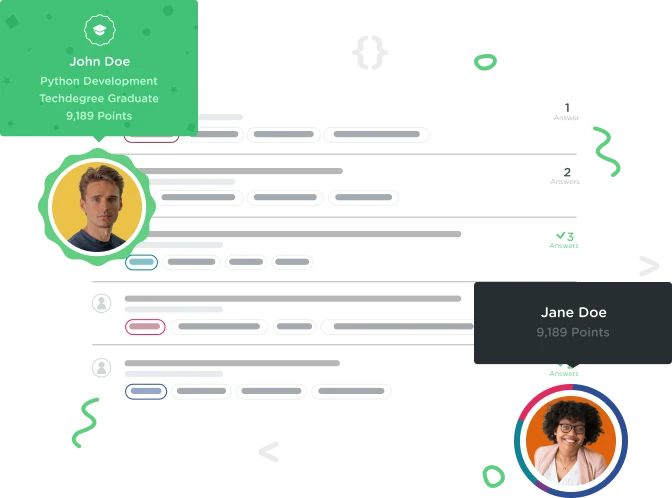

Daniel Wu
4,349 PointsWhy is my test failing?
from django.test import TestCase
from django.utils import timezone
from .models import Course, Step
class CourseModelTests(TestCase):
def test_course_creation(self):
course = Course.objects.create(
title="Python Regular Expressions",
description="Learn to write regular expressions in Python"
)
now = timezone.now()
self.assertLess(course.created_at, now)
class StepModelTests(TestCase):
def setUp(self):
self.course = Course.objects.create(
title="Python Testing",
description="Learn to write tests in Python"
)
def test_step_creation(self):
step = Step.objects.create(
title="Introduction to Doctests",
description="Learn to write tests in your docstrings.",
course=self.course
)
self.assertIn(step, self.course.step_set.all())
OUTPUT
python manage.py test
Creating test database for alias 'default'...
F.
======================================================================
FAIL: test_course_creation (courses.tests.CourseModelTests)
----------------------------------------------------------------------
Traceback (most recent call last):
File "C:\Users\AJ\learning_site2\courses\tests.py", line 14, in test_course_creation
self.assertLess(course.created_at, now)
AssertionError: datetime.datetime(2017, 3, 24, 5, 45, 9, 38206, tzinfo=<UTC>) not less than datetime.datetime(2017, 3, 24, 5, 45, 9, 38206, tzinfo=<UTC>)
----------------------------------------------------------------------
Ran 2 tests in 0.005s
FAILED (failures=1)
Destroying test database for alias 'default'...

Daniel Wu
4,349 PointsThank you Amin! Unfortunately I used AssertEqual and received a similar failure...
C:\Users\AJ\learning_site2>python manage.py test
Creating test database for alias 'default'...
F....
======================================================================
FAIL: test_course_creation (courses.tests.CourseModelTests)
----------------------------------------------------------------------
Traceback (most recent call last):
File "C:\Users\AJ\learning_site2\courses\tests.py", line 17, in test_course_creation
self.assertLess(course.created_at, now)
AssertionError: datetime.datetime(2017, 3, 24, 15, 8, 27, 867985, tzinfo=<UTC>) not less than datetime.datetime(2017, 3, 24, 15, 8, 27, 867985, tzinfo=<UTC>)
----------------------------------------------------------------------
Ran 5 tests in 0.060s
FAILED (failures=1)
Destroying test database for alias 'default'...
from django.core.urlresolvers import reverse
#get the name of the url, what's the route it goes to, and the view
from django.test import TestCase
from django.utils import timezone
from .models import Course, Step
class CourseModelTests(TestCase):
def test_course_creation(self):
course = Course.objects.create(
title="Python Regular Expressions",
description="Learn to write regular expressions in Python"
)
now = timezone.now()
self.assertEqual(course.created_at, now)
class StepModelTests(TestCase):
def setUp(self):
self.course = Course.objects.create(
title="Python Testing",
description="Learn to write tests in Python"
)
def test_step_creation(self):
step = Step.objects.create(
title="Introduction to Doctests",
description="Learn to write tests in your docstrings.",
course=self.course
)
self.assertIn(step, self.course.step_set.all())
class CourseViewsTests(TestCase):
def setUp(self):
#setup method to create things before writing tests
self.course = Course.objects.create(
title="Python Testing",
description="Learn to write tests in Python"
)
self.course2 = Course.objects.create(
title="New Course",
description="A new course"
)
self.step = Step.objects.create(
title="Introduction to Doctests",
description="Learn to write tests in your docstrings.",
course=self.course
)
def test_course_list_view(self):
resp = self.client.get(reverse('courses:list'))
#self.client is a webbrowser that makes web requests to the url
#and give you status code
#reverse the namespace list url
#make sure it's 200.
self.assertEqual(resp.status_code, 200)
self.assertIn(self.course, resp.context['courses'])
#make sure the views are in the contexts.
self.assertIn(self.course2, resp.context['courses'])
self.assertTemplateUsed(resp, 'courses/course_list.html')
self.assertContains(resp, self.course.title)
#makes sure the page contains the title
def test_course_detail_view(self):
resp = self.client.get(reverse('courses:detail',
kwargs={'pk': self.course.pk}))
self.assertEqual(resp.status_code, 200)
self.assertEqual(self.course, resp.context['course'])
def test_step_detail(self):
resp = self.client.get(reverse('courses:step', kwargs={
'course_pk': self.course.pk,
'step_pk': self.step.pk}))
self.assertEqual(resp.status_code, 200)
self.assertEqual(self.step, resp.context['step'])
5 Answers

Juan Ignacio Beloqui
3,064 PointsThe problem is that the record you have created and the variable you use to compare with are the same. It should be corrected in the videos i guess. I solved it like this:
self.assertLessEqual(course.created_at, now)
Look at the assert: is not assertLess but instead is an assertLessEqual which verifies if a is less or equal than b

Brady Rothrock
2,709 PointsI just used assertLess and was able to get it to work.
self.assertLess(course.created_at, now)
If you needed to use assertLessEqual then I wonder if it has to do with settings on individual machines or venvs of how precise the time values are stored.

Amin Ruhul
Courses Plus Student 4,762 PointsI guess error is different. You have some typo error
TypeError: unsupported operand type(s) for +: 'datetime.datetime' and 'int'
I recommend to have a look error and debug it.

Daniel Wu
4,349 PointsJust updated the correct error

Dillon Reyna
9,531 PointsSo the above code worked for me, but I have no idea why.
What is "step_set" and when did we make this?

Tanja Riet
10,803 PointsI would use import (from datetime import timedelta ) to be sure that there is a real time difference.
class CourseModelTests(TestCase): def test_course_creation(self): course = Course.objects.create(title='Python ',description='Learn to write regs') now = timezone.now() + timedelta(seconds=2) self.assertLess(course.created_at,now)

Amin Ruhul
Courses Plus Student 4,762 PointsYour error still saying your are using assertLess
``Traceback (most recent call last):
File "C:\Users\AJ\learning_site2\courses\tests.py", line 17, in test_course_creation
self.assertLess(course.created_at, now)
AssertionError: datetime.datetime(2017, 3, 24, 15, 8, 27, 867985, tzinfo=<UTC>) not less than datetime.datetime(2017, 3, 24, 15, 8, 27, 867985, tzinfo=<UTC>)
Amin Ruhul
Courses Plus Student 4,762 PointsAmin Ruhul
Courses Plus Student 4,762 PointsThe reason is you are created course and just taking that time as
created_at
and then comparing as less than to current time which is wrong.You can do in two ways: