Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial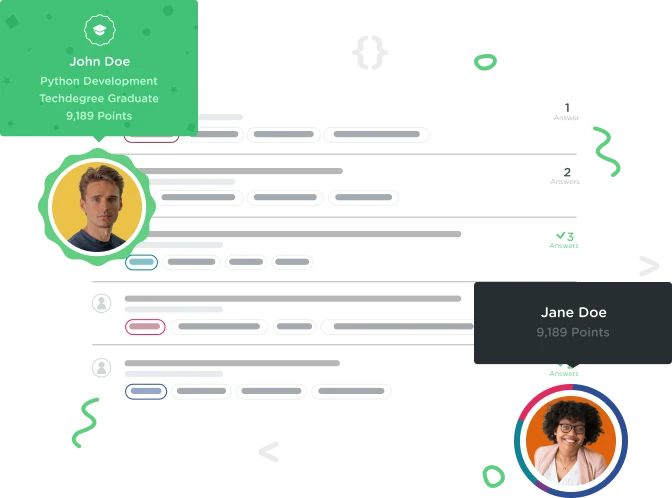

Alexander Joseph
2,014 PointsWhy is my UpdateView is showing as a DetailView? URL is correct when I click on edit button but shows a DetailView
URL is correct when I click on edit but the view looks the same as the DetailView - no form loads, just looks exactly the same as the DetailView. My CreateView works but UpdateView does not
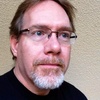
Chris Freeman
Treehouse Moderator 68,457 PointsCan you post your code?

Alexander Joseph
2,014 PointsThanks for your help, Here is my urls.py
from django.conf.urls import url, include
from . import views
urlpatterns = [
url(r'^$', views.NdaListView.as_view(), name='list'),
url(r'(?P<pk>\d+)/$', views.NdaDetailView.as_view(), name='detail'),
url(r'^create/$', views.NdaCreateView.as_view(), name='create'),
url(r'^edit/(?P<pk>\d+)/$', views.NdaUpdateView.as_view(), name='update'),
]
And here is my views.py
from django.shortcuts import get_object_or_404, render
from django.views.generic import (
ListView,
DetailView,
CreateView,
UpdateView,
DeleteView
)
from django.views.generic.edit import UpdateView
from .models import Nda
class NdaListView(ListView):
context_object_name = "ndas"
model = Nda
class NdaDetailView(DetailView):
model = Nda
class NdaCreateView(CreateView):
fields = ("disclosing_party", "disclosing_party_phone", "receiving_party", "receiving_party_phone", "date_effective_start", "date_effective_stop", "user")
model = Nda
class NdaUpdateView(UpdateView):
fields = ("disclosing_party", "disclosing_party_phone", "receiving_party", "receiving_party_phone", "date_effective_start", "date_effective_stop", "user")
model = Nda
my nda_form.html...
{% extends "layout.html" %}
{% block content %}
<h1>{% if not form.instance.pk %}Create NDA{% else %}Edit {{ form.instance.disclosing_party }}{% endif %}</h1>
<form method='POST'>
{% csrf_token %}
{{ form.as_p }}
<input type="submit" class="btn btn-primary" value="Save">
</form>
{% endblock %}
my nda_detail.html which has the link to the UpdateView...
{% extends "layout.html" %}
{% block title %}NDA Detail - {{ nda.disclosing_party }}{% endblock %}
{% block content %}
<h2>NDA Detail - {{ nda.disclosing_party }}</h2>
<table>
<tr>
<td>Disclosing Party: </td>
<td>{{ nda.disclosing_party }}</td>
</tr>
<tr>
<td>Disclosing Party Title: </td>
<td>{{ nda.disclosing_party_title }}</td>
</tr>
<tr>
<td>Disclosing Party Phone: </td>
<td>{{ nda.disclosing_party_phone }}</td>
</tr>
<tr>
<td>Disclosing Party Address: </td>
<td>{{ nda.disclosing_party_address }}</td>
</tr>
<tr>
<td>Disclosing Party City: </td>
<td>{{ nda.disclosing_party_city }}</td>
</tr>
<tr>
<td>Disclosing Party State: </td>
<td>{{ nda.disclosing_party_state }}</td>
</tr>
<tr>
<td>Disclosing Party Country: </td>
<td>{{ nda.disclosing_party_country }}</td>
</tr>
<tr>
<td>Requesting Party: </td>
<td>{{ nda.receiving_party }}</td>
</tr>
<tr>
<td>Requesting Party Title: </td>
<td>{{ nda.receiving_party_title }}</td>
</tr>
<tr>
<td>Requesting Party Phone: </td>
<td>{{ nda.receiving_party_phone }}</td>
</tr>
<tr>
<td>Requesting Party Address: </td>
<td>{{ nda.receiving_party_address }}</td>
</tr>
<tr>
<td>Requesting Party City: </td>
<td>{{ nda.receiving_party_city }}</td>
</tr>
<tr>
<td>Requesting Party State: </td>
<td>{{ nda.receiving_party_state }}</td>
</tr>
<tr>
<td>Requesting Party Country: </td>
<td>{{ nda.receiving_party_country }}</td>
</tr>
<tr>
<td>Requesting Party Country: </td>
<td>{{ nda.receiving_party_country }}</td>
</tr>
<tr>
<td>Effective: </td>
<td>{{ nda.date_effective_start }}</td>
</tr>
<tr>
<td>Expiration Date: </td>
<td>{{ nda.date_effective_start }}</td>
</tr>
<tr>
<td>NDA Notes: </td>
<td>{{ nda.notes|linebreaks }}</td>
</tr>
</table>
<hr />
<p><a href="{% url 'ndas:update' pk=nda.pk %}" class="btn">Edit</a></p>
{% endblock %}
And here is my models.py
from django.db import models
from django.core.urlresolvers import reverse
from django.contrib.auth.models import User
from django.core.urlresolvers import reverse
# Create your models here.
class Nda(models.Model):
created_at = models.DateTimeField(auto_now_add=True)
disclosing_party = models.CharField(max_length=255)
disclosing_party_title = models.CharField(max_length=255, blank=True, default='')
disclosing_party_phone = models.CharField(max_length=255)
disclosing_party_address = models.CharField(max_length=255, blank=True, default='')
disclosing_party_city = models.CharField(max_length=255, blank=True, default='')
disclosing_party_state = models.CharField(max_length=255, blank=True, default='')
disclosing_party_country = models.CharField(max_length=255, blank=True, default='')
receiving_party = models.CharField(max_length=255)
receiving_party_title = models.CharField(max_length=255, blank=True, default='')
receiving_party_phone = models.CharField(max_length=255)
receiving_party_address = models.CharField(max_length=255, blank=True, default='')
receiving_party_city = models.CharField(max_length=255, blank=True, default='')
receiving_party_state = models.CharField(max_length=255, blank=True, default='')
receiving_party_country = models.CharField(max_length=255, blank=True, default='')
date_effective_start = models.DateTimeField()
date_effective_stop = models.DateTimeField()
notes = models.TextField(blank=True, default='')
user = models.ForeignKey(User)
class Meta:
ordering = ['date_effective_start', ]
def __str__(self):
return self.disclosing_party
def get_absolute_url(self):
return reverse("ndas:detail", kwargs={"pk": self.pk})
2 Answers
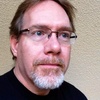
Chris Freeman
Treehouse Moderator 68,457 PointsDefinitely not an obvious error: missing leading carot (^) character in the DetailView url.
Without the ^ beginning-of-pattern marker, the DetailView URL also matches anything ending in digits, such as, "edit/4"
Thanks for the brain stretching question!! Post back if you need more help. Good luck!
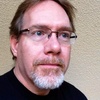
Chris Freeman
Treehouse Moderator 68,457 PointsI should add that if the edit
url was reordered to be before the detail
url, this latent error might not have been seen since urls work on a first match basis.

Alexander Joseph
2,014 PointsWow thanks very much. I need to get better at my regex!
Alexander Joseph
2,014 PointsAlexander Joseph
2,014 PointsActually when I click on edit the URL is correct but it just reloads the detailView, even the meta title is the same as the detail view