Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial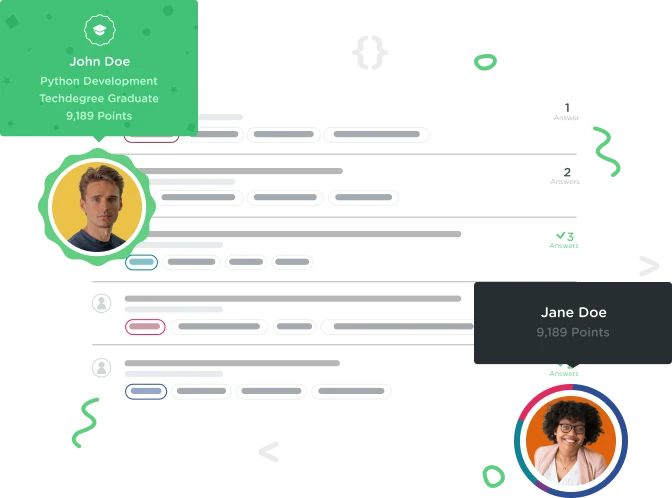

chloe shirk
878 Pointswhy is my while loop running print _ forever
I think the problem is with my second while loop. It keeps printing the _ after I press enter to run the game. I'm not sure how the condition isn't being met or where to add a break. I have tried changing the indentation of my else: and when I do the loop won't run at all.
i changed to second while loop to an if statement and it doesn't run forever but now I just guess a letter and it doesn't say if it's right or wrong and restarts the game with out counting strikes
import random
#make a list of words
words = [
'apple',
'banana',
'orange',
'coconut',
'strawberry',
'lime',
'grapefruit',
'lemon',
'kiwi',
'blueberry',
'melon'
]
while True:
start = input("Press enter/return to start, or Q to quit")
if start.lower() == 'q':
break
#pick rand word
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
#draw guessed letters, spaces, and strikes
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
#take guess
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You already guessed that letter!")
continue
elif not guess.isalpha():
print("You can only guess letters!")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You Win! The word was {}".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("You didn't get it! My secret word was {}".format(secret_word))
[MOD: added ```python formatting -cf]
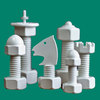
Steven Parker
231,268 PointsTry formatting your code so the spacing shows up (in Python, indentation is everything).
Use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.

chloe shirk
878 PointsSteven Parker thank you so much!
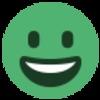
Jack Cummins
17,417 PointsI just want to ask, if you remember how many best answers I had, please tell me. I've been wanting to know for ages.
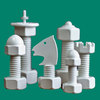
Steven Parker
231,268 PointsJack Cummins, I don't know any way to find that out except for when your name randomly shows up in the "Ask to Answer" section of a new question.
1 Answer
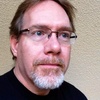
Chris Freeman
Treehouse Moderator 68,441 PointsEvery line from line 37 to the end of the code needs to indented one stop to function as part of the inner while
loop.
Also the line
If len(good_guesses) == len(list(secret_word)):
needs to be fixed. If the secret_word has any repeated letters, the number of correct guessed letters can not reach the number of secret word letters. Hint: how can you compare the number of guessed letters to the number of unique secret word letters.
chloe shirk
878 Pointschloe shirk
878 Pointshttps://stackoverflow.com/questions/50128111/why-is-my-while-loop-running-print-forever?noredirect=1#comment87274135_50128111