Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial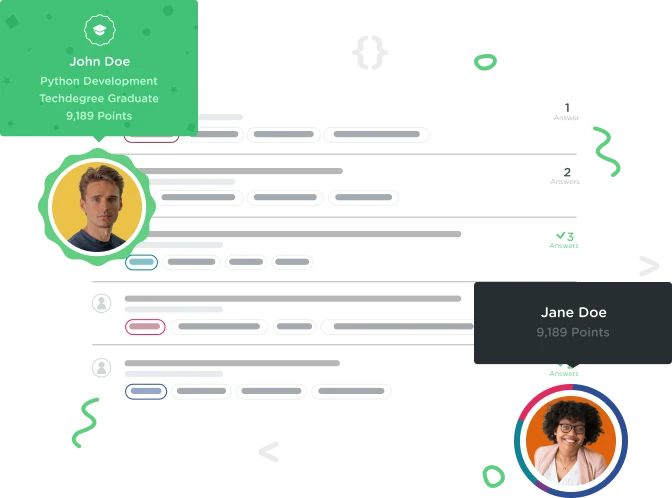

gaetan mertens
1,002 Pointswhy is "$" not a char?
I am trying to compare a variabel to the "$" symbol but it says that "$" is not a char. How do I compare it then?
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public boolean isAlpha(String text) {
char[] chars = text.toCharArray();
char a = "$";
for (char c : chars) {
if(Character.isLetter(c) || a==c ) {
return true;
}
}
return false;
}
private String normalizeDiscountCode(String discountCode) {
if (! isAlpha(discountCode)) {
throw new IlligalArgumentException("Invalid discount code");
}
discountCode = discountCode.toUpperCase();
return discountCode;
}
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
discountCode = normalizeDiscountCode(discountCode);
this.discountCode = discountCode;
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
1 Answer
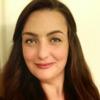
Jennifer Nordell
Treehouse TeacherHi there! First, the custom method you made isAlpha
is not required by the challenge, but above and beyond that you are taking a string and breaking it into an array of characters. The for loop you've constructed takes the first letter and assigns it to a local variable c
. Here's the tricky part. It's only ever going to evaluate the string up until something that counts as a character is true. The second a return statement is hit, the function ceases execution. If you were to send in "123abc", the function would end on "a" and return true. The only way it will return false is if it manages to get through the entire string and finds no characters.
My suggestion at this point, is to go back and reread the instructions of the challenge. Try not to implement any functions/methods that are not explicitly required by the challenge.
Hope this helps!
gaetan mertens
1,002 Pointsgaetan mertens
1,002 PointsThanks for your answer. So I have changed the code in the if statement but it still doesn't passes the code challenge. And besides, how am I suposed to verrify everry single char of a string without putting it in a loop and creating a new method for it?
Moderator edited: Added markdown so the code renders properly in the forums.
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherYour current code would work except for one teensy thing. The logic in your
if
statement is a bit off. You're saying if the character is not a letter or not a $, but you should be saying if the character is not a letter AND it is not a $ then return false. If I simply change your||
to an&&
, your code passes with flying colors!I had previously left a more explicit answer but removed it as your code works with just one tiny adjustment.
Hope this helps!