Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial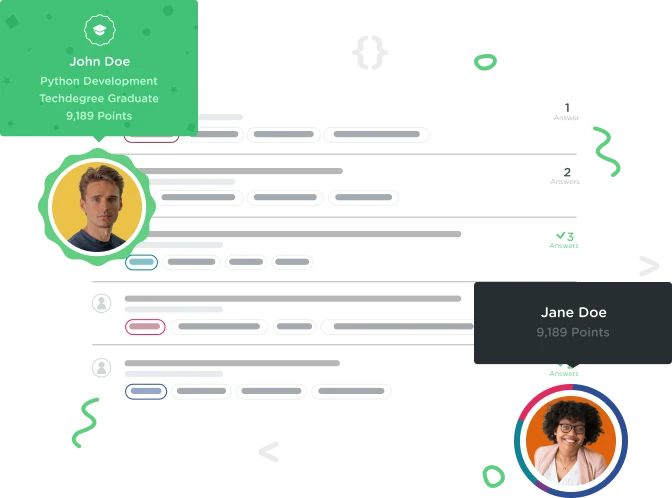

andres vargas
3,147 PointsWhy is not showing the error message??
function alea(n1,n2) { if( isNaN(n1) || isNaN(n2) ){ throw new Error('Ambos argumentos deben ser numeros'); } return Math.floor(Math.random() * (n2 - n1 + 1)) + n1; }
console.log(alea(40,50)); console.log(alea('9',11)); console.log(alea(50,60));

andres vargas
3,147 Pointshttps://w.trhou.se/2qmzb1c1pu here is my snapshot!!!!
2 Answers
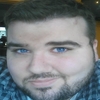
Marcus Parsons
15,719 PointsHey Andres,
The reason why the error is not thrown is because of the way isNaN
works. isNaN
converts strings into numbers and then tests them to see if they are not a number. So, the string '9' gets converted to a number and so isNaN
returns false. But if the string contains any non numerical characters isNaN
will be unable to convert the string into a number and isNaN
will return true.
Here is an article from the Mozilla Developer Network about isNaN: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/isNaN

andres vargas
3,147 Pointsso whats the solution? because i use the same code from the video (random number challenge, part II) as the teacher.
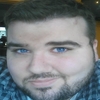
Marcus Parsons
15,719 PointsYou inputted the number 9 as a string, but the instructor spelled out the word nine. They are very different things, Andres.

andres vargas
3,147 Pointsohhhh, got it! Thank you very much :)
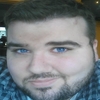
Marcus Parsons
15,719 PointsYou're welcome, Andres!
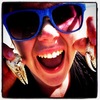
Allison Davis
12,698 PointsI think you need to wrap the second part of the function - return Math.floor(Math.random() * (n2 - n1 + 1)) + n1; - in an 'else' clause:
function alea(n1,n2) { if( isNaN(n1) || isNaN(n2) ){ throw new Error('Ambos argumentos deben ser numeros'); } else { return Math.floor(Math.random() * (n2 - n1 + 1)) + n1; } }
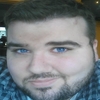
Marcus Parsons
15,719 PointsYou don't have to put an else clause here, because the return command only fires off if an error is not thrown.

andres vargas
3,147 Pointsnop, it doesnt work :(
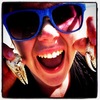
Allison Davis
12,698 PointsThanks, Marcus! :)
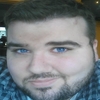
Marcus Parsons
15,719 PointsYou're welcome, Allison. Keep in mind that anything that stops execution of a script can be followed by an immediate return statement. For example, in the code below, the script will stop if the number 5 is an input in the function, but for every other number, it will return the number times 2. So, trying running this in your console:
function myfunc (num) {
if (parseInt(num) === 5) {
//returns always stop execution of the function
//you can also throw an error but that stops execution of the script
return false;
}
//notice no else clause here
return num * 2;
}
//will log false
console.log(myfunc(5));
//will log 4
console.log(myfunc(2));
//will log 8
console.log(myfunc(4));
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsHi Andres,
I tested your code in the console, and it works as it should. Can you please post a snapshot of your workspace for us to see? If you don't know how to do that, please check out this forum post: http://www.teamtreehouse.com/forum/workspace-snapshots