Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial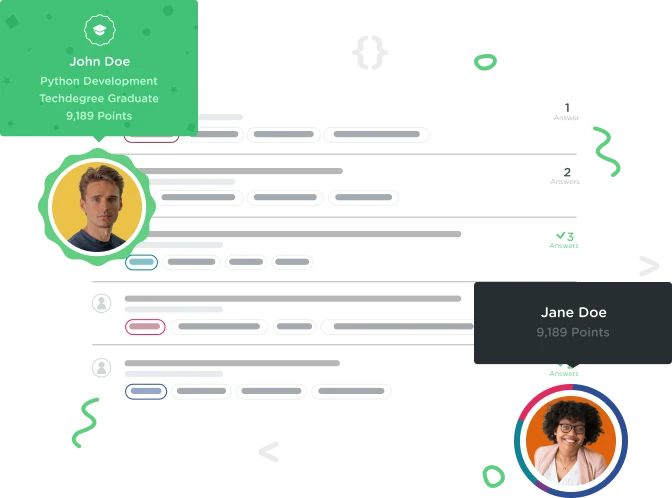

Ahmed SanaUllah
640 PointsWhy is response the parameter for .get() when the function expects a response back? Shouldn't it be req or request?
For the most part I have followed the course up until this point without a problem. However, one thing is confusing me, now that I have revisited the code after a short break. I don't understand why the https.get() function's 2nd parameter, response is called response. Isn't the get() function trying to return information using the response parameter? I think my confusion is not understanding where/how the response argument is being passed to .get(). Maybe I'm confusing a bigger concept with callback functions? Thank you for any clarification. :)
2 Answers

andren
28,558 PointsI do indeed think the issue is more your understanding of callback functions. A callback function is a function that is passed into another function. The get
method takes two parameters, the first is a URL which it should retrieve, and the second is a function it should execute after retrieving the URL.
The "parameter" you see in the get
method's argument list is not actually something that is passed to get
it is a parameter of the arrow function that you are passing into get
. In other words this entire block of code:
response => {
if (response.statusCode === 200) {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
try {
// Parse the data
const profile = JSON.parse(body);
// Print the data
printMessage(username, profile.badges.length, profile.points.JavaScript);
} catch (error) {
printError(error);
}
});
} else {
const message = `There was an error getting the profile for ${username} (${http.STATUS_CODES[response.statusCode]})`;
const statusCodeError = new Error(message);
printError(statusCodeError);
}
}
Is technically speaking one argument, the function that you are passing into get
. It might become easier to understand if the code is split into variables like this:
const url = `https://teamtreehouse.com/${username}.json`
// Create an arrow function that takes one parameter called response
const arrowFunction = response => {
if (response.statusCode === 200) {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
try {
// Parse the data
const profile = JSON.parse(body);
// Print the data
printMessage(username, profile.badges.length, profile.points.JavaScript);
} catch (error) {
printError(error);
}
});
} else {
const message = `There was an error getting the profile for ${username} (${http.STATUS_CODES[response.statusCode]})`;
const statusCodeError = new Error(message);
printError(statusCodeError);
}
}
// Notice that the arrowFunction is not called (no parenthesis is used) this is intentional
// as this is what tells JavaScript to just pass the function itself instead of calling it
const request = https.get(url, arrowFunction);
From that it should be more obvious that the parameter
and everything else following it is one value, which is passed into get
as an argument. The get
method is programmed to get the contents of the url passed to it and to call the function it was passed and pass the returned data in as the first argument. Some people have an easier time understanding this when a regular function is being used instead of an arrow function, so here is an example of the code without any arrow functions:
const request = https.get(`https://teamtreehouse.com/${username}.json`, function(response) {
if (response.statusCode === 200) {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
try {
// Parse the data
const profile = JSON.parse(body);
// Print the data
printMessage(username, profile.badges.length, profile.points.JavaScript);
} catch (error) {
printError(error);
}
});
} else {
const message = `There was an error getting the profile for ${username} (${http.STATUS_CODES[response.statusCode]})`;
const statusCodeError = new Error(message);
printError(statusCodeError);
}
});
So to reiterate the response
parameter belongs to the function you pass into get
, and it is named response
because the get
method passes the response it gets as the first argument to the function that is passed to it. Callback functions are a thing that often causes confusing among new programmers, as it is the first example they usually come across of a function itself being passed as a value, rather than passing the return of a function which is far more common.
The important think to keep in mind is that in JavaScript functions are technically speaking no different than strings or numbers, in terms of just being a basic value that can be assigned to a variable and passed around freely from function to function.

Rijio Rifat
83 Pointsimport json def json_to(anything): return json.dumps(anything)
Ahmed SanaUllah
640 PointsAhmed SanaUllah
640 PointsThank you so much! That explanation nailed it. :)