Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial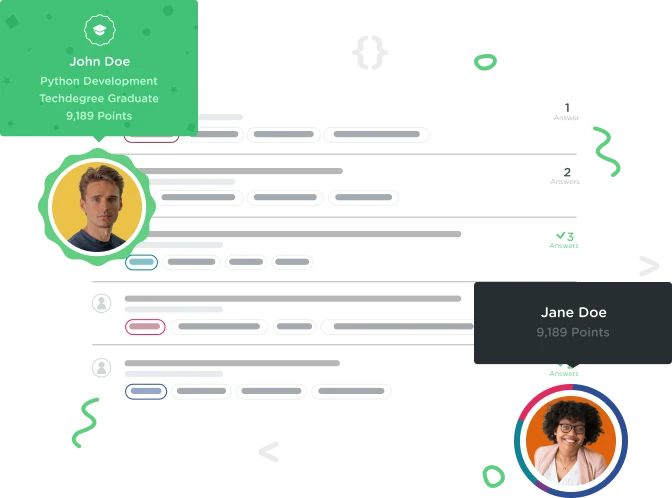

James Adamski
3,027 PointsWhy is Task 1 failing after passing tests
The code below is step 2 of this challenge is causing Task 1 to fail. I have been back and forth for a couple of days and don't see why. Can you spot the problem? Going back and changing the methods on TASK 1 does not help. I have several iterations of this code and the only one that passed failed to do the validation of the discountCode. I am not sure what the problem is and could use some help.
public class Order { private String itemName; private int priceInCents; private String discountCode;
public Order(String itemName, int priceInCents) { this.itemName = itemName; this.priceInCents = priceInCents; }
public String getItemName() { return itemName; }
public int getPriceInCents() { return priceInCents; }
public String getDiscountCode() { return discountCode; }
public void applyDiscountCode(String discountCode) {
try {
this.discountCode = normalizeDiscountCode(discountCode);
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage());
}
}
private String normalizeDiscountCode(String discountCode) { for (char ch : discountCode.toCharArray()) { if( ! Character.isLetter(ch) || Character.isDigit(ch) || Character.getType(ch) != 26 ){ throw new IllegalArgumentException("Invalid discount code"); } } return discountCode.toUpperCase(); } }
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
try {
this.discountCode = normalizeDiscountCode(discountCode);
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage());
}
}
private String normalizeDiscountCode(String discountCode) {
for (char ch : discountCode.toCharArray()) {
if( ! Character.isLetter(ch) || Character.isDigit(ch) || Character.getType(ch) != 26 ){
throw new IllegalArgumentException("Invalid discount code");
}
}
return discountCode.toUpperCase();
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
2 Answers

Ryan S
27,276 PointsHi James,
You are on the way to a good solution and really close, but there are just a couple issues with your code.
The first has to do with the if
condition. When using a combination of "not" operators and or
conditions, things can get confusing pretty quick. It took me a while to track down the issue.
The way you have it now, the check is failing on the last or
condition, Character.getType(ch) != 26
.
Say you had a valid letter passed into the condition. The first condition would return false, the second would return false, but the last would return true, since although it is a letter and should be valid, it is not a dollar sign.
To fix this, you can group the isLetter and getType conditions together:
for (char ch : discountCode.toCharArray()) {
if ( !(Character.isLetter(ch) || Character.getType(ch) == 26) || Character.isDigit(ch) ){
throw new IllegalArgumentException("Invalid discount code");
}
}
The second issue is in the "applyDiscountCode" method. The challenge is expecting an Exception to be thrown, as shown in Example.java, but you are handling this before it can be caught by the outer code. You'll need to remove the try/catch blocks in order for it to pass.
Hope this clears things up.

James Adamski
3,027 PointsThanks! A little frustrating to know that I was so close and had too much code and not too little. I got it two work this time. I am sure I will have more questions later.