Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial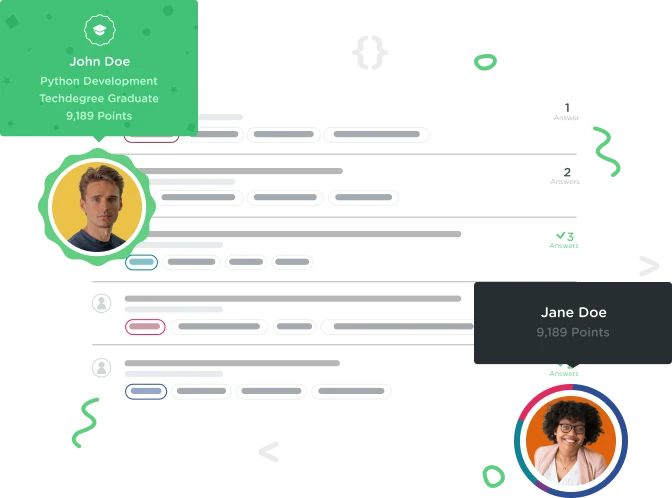

Brandon Scott
8,355 PointsWhy is the code not able to recognize the console object
I'm not sure if I'm even going the right direction with this code any advice would be helpful
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
int line = 0;
Console console = System.console();
String enterLastName = console.readLine("Enter last name: ");
char name = enterLastNameAsString.charAt(0);
if (name > 'm') {
line = 2;
} else {
line = 1;
}
return line;
}
}
3 Answers
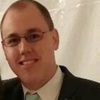
Jeremiah Montano
7,221 PointsThe compiler is not reading the console object because you did not import it. You also do not need to import a console class because you already have a last name to test. The method takes in a variable of lastName so you do not need the user to provide another last name.
Also remember that lowercase does not equal Uppercase. The comment lines indicate you will be comparing uppercase letters so make sure your if statement contains an uppercase letter as well.
Also, there is a variable that is called but never initialized, "enterLastNameAsString." This variable should be changed to match the variable that is created upon method initialization, or lastName.
I hope this helps. Please let me know if you have any other questions. Happy coding!

Brandon Scott
8,355 PointsWow you helped a lot thaks.
I'm curios though how would I check for a uppercase letter as well. I know we used something in earlier to check both lower and capital letters but how would I use charAt and check for both lower and capital letters.
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
int line = 0;
char name = lastName.charAt(0);
if (name > 'M') {
line = 2;
} else {
line = 1;
}
return line;
}
}
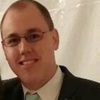
Jeremiah Montano
7,221 PointsIf you wanted to check for uppercase or lowercase, you would use Character.isUpperCase(char) method. Also, you can use toUpperCase to ensure validity. So in your code, to ensure the right line is picked you could use:
if (Character.toUpperCase(name) > 'M') {
//statement code
}
This would ensure, no matter what, you were always comparing an uppercase letter to an uppercase letter. The java documentation linked below is helpful for knowing what methods the Character class already has:

Brandon Scott
8,355 PointsUnderstood, I guess I thought there was a way to do it on one line. I feel I'm starting to understand this java thing a little bit better. Thanks for your help again.