Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial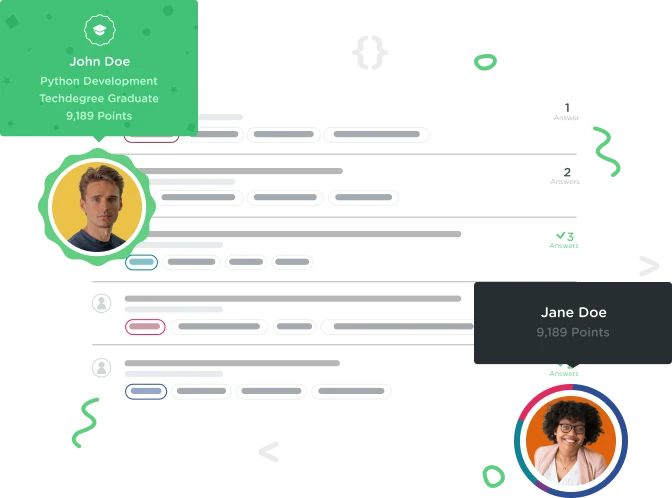

Reagan Kambayi
358 PointsWhy is the data from my program not being saved on SQL lite?
I am creating a program that holds details about booking a hotel, when the user enters the detail the information is not saved in the SQL Lite. What should I do?
import modules
from tkinter import *
tkinter is a module in python and this means import everything from tkinter which is used to create a GUI graphical user interface
import sqlite3 import tkinter.messagebox
This is a module that allows you to connect to the SQL database
This will allow the computer to create a mespythosagebox
connect to the database
conn = sqlite3.connect('database.db')
cursor to move around the database
c = conn.cursor() print("Successfully connected")
tkinter window
class Application: def init(self, master): self.master = master
# creating the frames in the master
self.left = Frame(master, width=800, height=720, bg='black')
self.left.pack(side=LEFT)
self.right = Frame(master, width=400, height=720, bg='navyblue')
self.right.pack(side=RIGHT)
# labels for the window
self.heading = Label(self.left, text="Bienvenue a l'hotel De Kambayi ", font=('arial 40 bold'), fg='white', bg='black')
self.heading.place(x=0, y=0)
# customer name
self.name = Label(self.left, text="Name", font=('arial 18 bold'), fg='white', bg='black')
self.name.place(x=0, y=100)
# age
self.age = Label(self.left, text="Age", font=('arial 18 bold'), fg='white', bg='black')
self.age.place(x=0, y=150)
# gender
self.gender = Label(self.left, text="Gender", font=('arial 18 bold'), fg='white', bg='black')
self.gender.place(x=0, y=200)
# room
self.room = Label(self.left, text="Room", font=('arial 18 bold'), fg='white', bg='black')
self.room.place(x=0, y=250)
# phone
self.phone = Label(self.left, text="Phone", font=('arial 18 bold'), fg='white', bg='black')
self.phone.place(x=0, y=300)
# scheduled_time
self.scheduledtime = Label(self.left, text="Scheduled-time", font=('arial 18 bold'), fg='white', bg='black')
self.scheduledtime.place(x=0, y=350)
# Entries for all labels
self.name_ent = Entry(self.left, width=30)
self.name_ent.place(x=250, y=100)
self.gender_ent = Entry(self.left, width=30)
self.gender_ent.place(x=250, y=150)
self.age_ent = Entry(self.left, width=30)
self.age_ent.place(x=250, y=200)
self.room_ent = Entry(self.left, width=30)
self.room_ent.place(x=250, y=250)
self.phone_ent = Entry(self.left, width=30)
self.phone_ent.place(x=250, y=300)
self.scheduledtime_ent = Entry(self.left, width=30)
self.scheduledtime_ent.place(x=250, y=350)
# button to perform a command
self.submit = Button(self.left, text="Book now at l'hotel De Kambayi", width=25, height=2, bg='blue')
self.submit.place(x=300, y=500)
# function to call when the submit button is clicked
def add_appointment(self):
# getting the user inputs
self.val1 = self.name_ent.get()
self.val2 = self.age_ent.get()
self.val3 = self.gender_ent.get()
self.val4 = self.room_ent.get()
self.val5 = self.phone_ent.get()
self.val6 = self.scheduled_time_ent.get()
# checking if the user input is empty
if self.val1 == '' or self.val2 == '' or self.val3 == '' or self.val4 == '' or self.val5 == '' or self.val6 == '':
tkinter.messagebox.showinfo("Warning", "Please Fill Up All The Boxes")
else:
# now we add to the database
sql = "INSERT INTO 'appointments' (name, age, gender, room, phone, scheduled_time) VALUES(?, ?, ?, ?, ?, ?)"
c.execute(sql, (self.val1, self.val2, self.val3, self.val4, self.val5, self.val6))
conn.commit()
c.close()
conn.close()
print("Successfully added to the database")
creating the object
root = Tk() root.title('Kambayi') b = Application(root)
resolution of the window
root.geometry("1200x720+0+0")
preventing the resize feature
root.resizable(False, False)
end the loop
root.mainloop()