Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial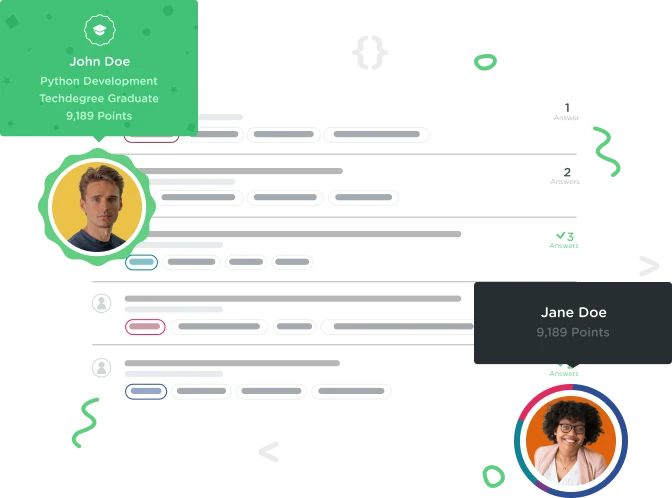

Vlatko .
2,526 PointsWhy is the function written twice like this?
This is how it's written in the tutorial:
int funky_math(int a, int b);
int main() {
int foo = 24;
int bar = 35;
printf("funky math %d", funky_math(foo, bar));
return 0;
}
int funky_math(int a, int b) {
return a + b + 343;
}
What has me confused is, what does the function funky_math in the topmost line do? Why is it written this way? I have written it the way below and it works as well, but am wondering what the difference between the two is.
My version:
int funky_math(int a, int b) {
return a + b + 343;
}
int main() {
int foo = 24;
int bar = 35;
printf("funky math %d", funky_math(foo, bar));
return 0;
}
Thanks.
3 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Vlatko,
In your first code block, that first line is the function declaration. Then at the bottom where you've written the code for the function is called the function definition. By placing the declaration at the top, it allows you to call the function before you've actually defined it. Notice that you were able to call the function inside main
before the function was defined. So using a function declaration at the top gives you flexibility in where you can place your function definitions. You can put them all at the bottom of your file so that they're out of the way and you can have your main
function near the top.
If you don't use a function declaration as in your second code block then you have to make sure you put the function definition before you try to call the function.
Let me know if there's anything that still isn't clear.

Michael Wiedenheft
229 PointsIs one better practice than the other?

Jason Anello
Courses Plus Student 94,610 PointsHi Michael,
I think more people would prefer putting the function declarations at the top and then the function definitions after main
.
It's mainly an organization thing. It doesn't seem to make much difference here because there's only 1 extra function here, in addition to main
, and it's a short 1 liner.
Imagine instead you had 15 functions all with 10 to 15 lines of code each. You could either have 15 lines of function declarations at the top with your main function right after that. Or you could have several hundred lines of function definitions before you get to the main function.

Vlatko .
2,526 Points.
Vlatko .
2,526 PointsVlatko .
2,526 PointsAhhh, alright. So it's more of an organizational practice. In my little experience, I've always written functions before they are called, so this will help out in terms of keeping things more organized. Thanks for the help.